功能:N名学生的成绩已在主函数中放入一个带头节点的链表结构中,h指向链表的头节点。请编写子函数double fun(STREC *h),找出学生的最高分,由函数值返回。 说明,链表的创立建议另定义一子函数实现,并在主函数中调用实现。 注意:链表的节点类型定义如下: struct slist { double s; struct slist *next; }; typedef struct slist STREC;
时间: 2024-03-09 13:46:34 浏览: 84
好的,我理解了你的问题。下面是实现该功能的代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct slist {
double s;
struct slist *next;
} STREC;
void createList(STREC **h, int n) {
*h = (STREC *) malloc(sizeof(STREC));
(*h)->next = NULL;
STREC *p = *h;
int i;
for (i = 0; i < n; i++) {
STREC *node = (STREC *) malloc(sizeof(STREC));
scanf("%lf", &(node->s));
node->next = NULL;
p->next = node;
p = node;
}
}
double fun(STREC *h) {
double max = 0.0;
STREC *p = h->next;
while (p != NULL) {
if (p->s > max) {
max = p->s;
}
p = p->next;
}
return max;
}
int main() {
int n;
printf("请输入学生人数:");
scanf("%d", &n);
STREC *h;
createList(&h, n);
double max = fun(h);
printf("最高分为:%.2f\n", max);
STREC *p = h->next, *q;
while (p != NULL) {
q = p->next;
free(p);
p = q;
}
free(h);
return 0;
}
```
在该代码中,我们首先定义了一个 `STREC` 结构体,表示每个学生的成绩。然后我们编写了一个 `createList` 函数,该函数的输入参数为指向 `STREC` 结构体的二级指针 `h` 和整型变量 `n`,作用是动态创建一个带头节点的链表,并将链表头节点的地址保存在 `h` 中。接着我们编写了一个 `fun` 函数,该函数的输入参数为指向头节点的指针 `h`,输出参数为最高分。该函数的作用是遍历链表,找出最高分并返回。
在 `main` 函数中,我们首先读入学生人数,然后调用 `createList` 函数创建带头节点的链表并读入每个学生的成绩。接着调用 `fun` 函数计算最高分并输出。最后别忘了释放动态分配的内存。
阅读全文
相关推荐
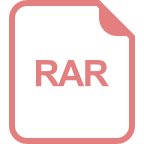
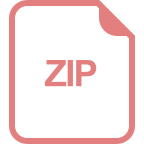
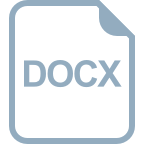
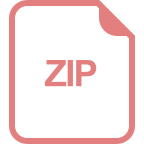
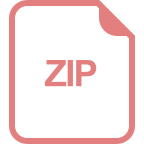
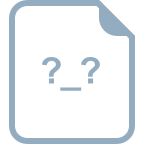
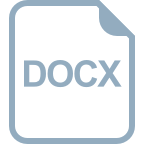
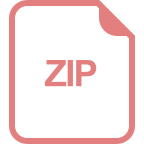