如何从QDateTime类中获取当日积秒
时间: 2024-04-08 22:29:17 浏览: 90
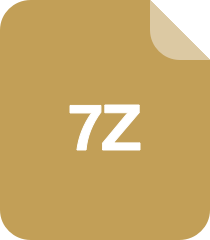
Qt例程源代码QDateTime.7z

要从`QDateTime`类中获取当日的累计秒数,你可以使用`QDateTime::time()`方法来获取时间部分,然后使用`QTime::msecsSinceStartOfDay()`方法来获取从当天开始到现在的毫秒数,最后将其转换为秒数。
下面是一个示例代码:
```cpp
QDateTime qdTime = QDateTime::currentDateTime();
QTime qTime = qdTime.time();
int seconds = qTime.msecsSinceStartOfDay() / 1000;
```
在上述代码中,我们首先使用`QDateTime::currentDateTime()`来获取当前日期和时间。然后,通过调用`time()`方法,我们可以从`QDateTime`对象中获取时间部分,并将其赋值给`qTime`变量。
接下来,我们使用`msecsSinceStartOfDay()`方法来获取从当天开始到现在的毫秒数。最后,我们将得到的毫秒数除以1000,将其转换为秒数,并将结果赋值给`seconds`变量。
这样,`seconds`变量将包含当日的累计秒数。你可以继续使用`seconds`变量进行后续的操作和处理。
阅读全文
相关推荐
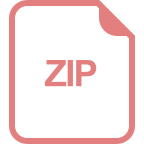
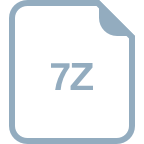















