python展示心形代码的五种方法
时间: 2023-11-12 11:13:17 浏览: 51
以下是五种Python展示心形的代码方法:
方法1:
```
import turtle
t = turtle.Turtle()
t.penup()
t.goto(0, -200)
t.pendown()
t.begin_fill()
t.color('red')
for x in range(150):
if x < 75:
t.forward(2)
t.right(1)
else:
t.forward(2)
t.left(1)
t.end_fill()
turtle.done()
```
方法2:
```
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(-2, 2, 500)
y1 = np.sqrt(1 - (np.abs(x) - 1) ** 2)
y2 = -3 * np.sqrt(1 - (np.abs(x) / 2) ** 0.5)
plt.fill_between(x, y1, color='red')
plt.fill_between(x, y2, color='red')
plt.xlim([-2.5, 2.5])
plt.ylim([-3, 2])
plt.axis('off')
plt.show()
```
方法3:
```
import numpy as np
import matplotlib.pyplot as plt
def f(x, y):
return ((x ** 2 + y ** 2 - 1) ** 3 - x ** 2 * y ** 3)
x, y = np.mgrid[-2:2:100j, -2:2:100j]
z = f(x, y)
plt.contour(x, y, z, levels=[0], colors=['red'])
plt.axis('off')
plt.show()
```
方法4:
```
import turtle
t = turtle.Turtle()
t.hideturtle()
t.getscreen().bgcolor("black")
t.color("red")
for i in range(200):
t.speed(0)
t.right(i)
t.forward(i * 2)
turtle.done()
```
方法5:
```
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(-2, 2, 500)
y1 = np.sqrt(1 - (np.abs(x) - 1) ** 2)
y2 = -3 * np.sqrt(1 - (np.abs(x) / 2) ** 0.5)
plt.plot(x, y1, color='red')
plt.plot(x, y2, color='red')
plt.xlim([-2.5, 2.5])
plt.ylim([-3, 2])
plt.axis('off')
plt.show()
```
以上是五种Python展示心形的代码方法,每种方法都有其独特的优点和适用场景。
阅读全文
相关推荐
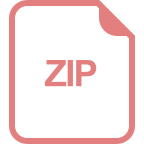
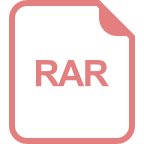
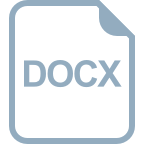















