linux c++ 扫描sd卡硬盘剩余容量, 剩余容量低于10%的话,删除最早的文件,直到剩余容量高于15%
时间: 2023-06-19 22:03:39 浏览: 291
你可以使用Linux系统的statfs函数来获取文件系统的信息,包括总容量、已用空间和剩余空间等。以下是一个简单的示例代码:
```c++
#include <sys/vfs.h>
#include <iostream>
int main() {
struct statfs diskInfo;
if (statfs("/mnt/sdcard", &diskInfo) == -1) {
std::cerr << "Failed to get disk info" << std::endl;
return -1;
}
unsigned long long totalBytes = diskInfo.f_blocks * diskInfo.f_bsize;
unsigned long long freeBytes = diskInfo.f_bfree * diskInfo.f_bsize;
unsigned long long usedBytes = totalBytes - freeBytes;
double freePercent = (double)freeBytes / totalBytes * 100;
double usedPercent = (double)usedBytes / totalBytes * 100;
std::cout << "Total capacity: " << totalBytes / (1024*1024) << " MB" << std::endl;
std::cout << "Used space: " << usedBytes / (1024*1024) << " MB (" << usedPercent << "%)" << std::endl;
std::cout << "Free space: " << freeBytes / (1024*1024) << " MB (" << freePercent << "%)" << std::endl;
return 0;
}
```
你可以使用一个while循环,不断获取剩余空间并检查是否需要删除文件。如果剩余容量低于10%,则删除最早的文件,直到剩余容量高于15%。以下是一个示例代码:
```c++
#include <sys/vfs.h>
#include <sys/stat.h>
#include <dirent.h>
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
const std::string ROOT_DIR = "/mnt/sdcard";
const double MIN_FREE_PERCENT = 10.0;
const double MAX_FREE_PERCENT = 15.0;
const int MAX_FILES_TO_DELETE = 10;
bool isDirectory(const std::string& path) {
struct stat s;
if (stat(path.c_str(), &s) == 0) {
return S_ISDIR(s.st_mode);
}
return false;
}
void listFiles(const std::string& path, std::vector<std::string>& files) {
DIR* dir = opendir(path.c_str());
if (dir == nullptr) {
return;
}
struct dirent* entry;
while ((entry = readdir(dir)) != nullptr) {
if (entry->d_name[0] == '.') {
continue;
}
std::string fullPath = path + "/" + entry->d_name;
if (isDirectory(fullPath)) {
listFiles(fullPath, files);
} else {
files.push_back(fullPath);
}
}
closedir(dir);
}
bool sortByLastModifiedTime(const std::string& a, const std::string& b) {
struct stat s1, s2;
if (stat(a.c_str(), &s1) == -1 || stat(b.c_str(), &s2) == -1) {
return false;
}
return s1.st_mtime < s2.st_mtime;
}
bool deleteFile(const std::string& path) {
return remove(path.c_str()) == 0;
}
int main() {
while (true) {
struct statfs diskInfo;
if (statfs(ROOT_DIR.c_str(), &diskInfo) == -1) {
std::cerr << "Failed to get disk info" << std::endl;
break;
}
unsigned long long totalBytes = diskInfo.f_blocks * diskInfo.f_bsize;
unsigned long long freeBytes = diskInfo.f_bfree * diskInfo.f_bsize;
double freePercent = (double)freeBytes / totalBytes * 100;
if (freePercent > MAX_FREE_PERCENT) {
break;
std::vector<std::string> files;
listFiles(ROOT_DIR, files);
std::sort(files.begin(), files.end(), sortByLastModifiedTime);
int numFilesToDelete = std::min((int)files.size(), MAX_FILES_TO_DELETE);
for (int i = 0; i < numFilesToDelete; i++) {
if (!deleteFile(files[i])) {
std::cerr << "Failed to delete file: " << files[i] << std::endl;
}
freeBytes += fileSize(files[i]);
freePercent = (double)freeBytes / totalBytes * 100;
if (freePercent > MAX_FREE_PERCENT) {
break;
}
}
}
return 0;
}
```
在该示中,我们首先使用isDirectory函数判断一个路径是否为目录,如果目录则递归调用listFiles函数获取目录下的所有文件,并将它们存储在一个vector中。然后我们使用sortByLastModifiedTime函数将文件按照最后修改时间排序,从而删除最早的文件。最后我们使用deleteFile函数删除文件,并将释放的空间加入到剩余空间中。
阅读全文
相关推荐







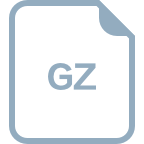
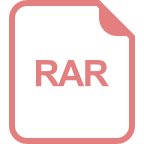






