用springboot,vue,mybatis和elementUI实现上传PDF文件到数据库,需要上传到数据库的内容有文件名,文件上传时间,文件路径,上传者的编号。请给我domain代码,controller代码,业务逻辑组件类代码,前端vue和element代码,mybatis代码和数据库建表语句,并用注释讲解
时间: 2023-05-20 18:06:01 浏览: 67
非常感谢您的问题,以下是您所需要的代码和注释:
1. 首先,我们需要创建一个domain类来存储上传的PDF文件的信息,包括文件名,文件上传时间,文件路径和上传者的编号。我们可以创建一个名为PdfFile的类:
```java
public class PdfFile {
private Long id; // 文件id
private String fileName; // 文件名
private String filePath; // 文件路径
private Date uploadTime; // 文件上传时间
private Long uploaderId; // 上传者编号
// getter和setter方法
}
```
2. 接下来,我们需要创建一个controller类来处理上传文件的请求。我们可以创建一个名为PdfFileController的类:
```java
@RestController
@RequestMapping("/pdf")
public class PdfFileController {
@Autowired
private PdfFileService pdfFileService;
@PostMapping("/upload")
public Result upload(@RequestParam("file") MultipartFile file, Long uploaderId) {
try {
PdfFile pdfFile = pdfFileService.save(file, uploaderId);
return Result.success(pdfFile);
} catch (Exception e) {
e.printStackTrace();
return Result.error("上传失败");
}
}
}
```
3. 然后,我们需要创建一个业务逻辑组件类来处理上传文件的业务逻辑。我们可以创建一个名为PdfFileService的类:
```java
@Service
public class PdfFileService {
@Autowired
private PdfFileMapper pdfFileMapper;
public PdfFile save(MultipartFile file, Long uploaderId) throws IOException {
String fileName = file.getOriginalFilename();
String filePath = "pdf/" + UUID.randomUUID().toString() + fileName.substring(fileName.lastIndexOf("."));
File dest = new File(filePath);
file.transferTo(dest);
PdfFile pdfFile = new PdfFile();
pdfFile.setFileName(fileName);
pdfFile.setFilePath(filePath);
pdfFile.setUploadTime(new Date());
pdfFile.setUploaderId(uploaderId);
pdfFileMapper.insert(pdfFile);
return pdfFile;
}
}
```
4. 接下来,我们需要创建前端vue和element代码来实现上传文件的功能。我们可以创建一个名为PdfFileUpload的组件:
```vue
<template>
<div>
<el-upload
class="upload-demo"
action="/pdf/upload"
:data="{ uploaderId: uploaderId }"
:on-success="handleSuccess"
:before-upload="beforeUpload"
:show-file-list="false"
>
<el-button size="small" type="primary">点击上传</el-button>
</el-upload>
</div>
</template>
<script>
export default {
name: "PdfFileUpload",
props: {
uploaderId: {
type: Number,
required: true,
},
},
methods: {
beforeUpload(file) {
const isPdf = file.type === "application/pdf";
if (!isPdf) {
this.$message.error("只能上传PDF文件");
}
return isPdf;
},
handleSuccess(response) {
this.$emit("success", response.data);
},
},
};
</script>
```
5. 然后,我们需要创建mybatis代码来实现对数据库的操作。我们可以创建一个名为PdfFileMapper的接口:
```java
@Mapper
public interface PdfFileMapper {
@Insert("INSERT INTO pdf_file(file_name, file_path, upload_time, uploader_id) VALUES(#{fileName}, #{filePath}, #{uploadTime}, #{uploaderId})")
@Options(useGeneratedKeys = true, keyProperty = "id")
int insert(PdfFile pdfFile);
}
```
6. 最后,我们需要创建数据库建表语句来创建pdf_file表:
```sql
CREATE TABLE `pdf_file` (
`id` bigint(20) NOT NULL AUTO_INCREMENT COMMENT '文件id',
`file_name` varchar(255) NOT NULL COMMENT '文件名',
`file_path` varchar(255) NOT NULL COMMENT '文件路径',
`upload_time` datetime NOT NULL COMMENT '文件上传时间',
`uploader_id` bigint(20) NOT NULL COMMENT '上传者编号',
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COMMENT='PDF文件表';
```
以上就是使用springboot,vue,mybatis和elementUI实现上传PDF文件到数据库的代码和注释,希望能够帮助到您。
相关推荐
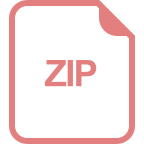
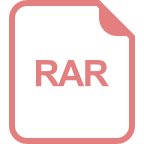


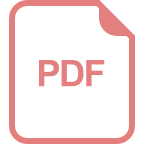
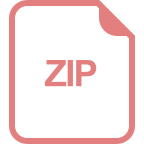
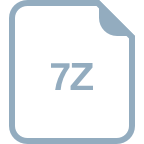
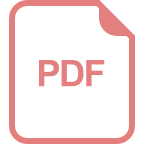
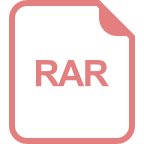
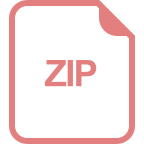
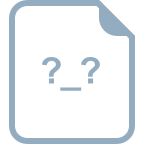
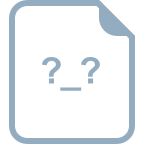
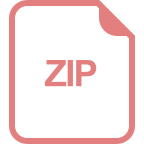