用C语言写一个简易的商品管理系统
时间: 2024-09-12 09:15:19 浏览: 44
一个简易的商品管理系统通常包含商品信息的添加、删除、修改和查询等基本功能。下面是一个基于C语言的简易商品管理系统的简单示例,包含商品结构体定义和简单的功能实现。
首先定义一个商品结构体,用于存储商品信息:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 商品信息结构体
typedef struct {
int id; // 商品ID
char name[50]; // 商品名称
float price; // 商品价格
int quantity; // 商品库存量
} Product;
// 商品列表,用于存储多个商品信息
#define MAX_PRODUCTS 100
Product products[MAX_PRODUCTS];
int productCount = 0; // 当前商品数量
// 函数声明
void addProduct(int id, const char* name, float price, int quantity);
void deleteProduct(int id);
void updateProduct(int id, const char* name, float price, int quantity);
Product* getProduct(int id);
void listProducts();
```
以下是商品管理系统的简单功能实现:
```c
// 添加商品
void addProduct(int id, const char* name, float price, int quantity) {
if (productCount < MAX_PRODUCTS) {
products[productCount].id = id;
strcpy(products[productCount].name, name);
products[productCount].price = price;
products[productCount].quantity = quantity;
productCount++;
} else {
printf("商品数量已达上限,无法添加新商品。\n");
}
}
// 删除商品
void deleteProduct(int id) {
int i;
for (i = 0; i < productCount; i++) {
if (products[i].id == id) {
for (int j = i; j < productCount - 1; j++) {
products[j] = products[j + 1];
}
productCount--;
return;
}
}
printf("未找到ID为%d的商品。\n", id);
}
// 更新商品信息
void updateProduct(int id, const char* name, float price, int quantity) {
Product* p = getProduct(id);
if (p != NULL) {
strcpy(p->name, name);
p->price = price;
p->quantity = quantity;
} else {
printf("未找到ID为%d的商品。\n", id);
}
}
// 获取商品信息
Product* getProduct(int id) {
for (int i = 0; i < productCount; i++) {
if (products[i].id == id) {
return &products[i];
}
}
return NULL;
}
// 列出所有商品信息
void listProducts() {
for (int i = 0; i < productCount; i++) {
printf("ID: %d, 名称: %s, 价格: %.2f, 数量: %d\n",
products[i].id, products[i].name, products[i].price, products[i].quantity);
}
}
```
在这个示例中,我们定义了商品信息结构体`Product`,一个能够存储最多100个商品的数组`products`,以及一个记录当前商品数量的变量`productCount`。我们提供了添加、删除、更新和列出商品信息的基本函数。`getProduct`函数用于通过商品ID获取商品指针,如果不存在则返回`NULL`。
请注意,上述代码是一个框架性质的示例,它没有包含完整的用户界面和数据持久化部分,实际使用时需要根据具体需求进行完善和扩展。
阅读全文
相关推荐
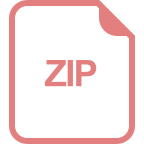
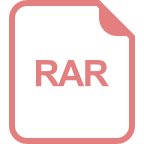







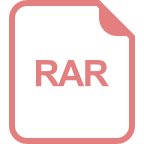







