创建线程安全的单例有那些实现⽅法?
时间: 2024-03-09 09:49:30 浏览: 15
创建线程安全的单例有以下几种实现方法:
1. 饿汉式单例模式
在类加载时即创建单例对象,线程安全,但可能会浪费一些内存空间。
```java
public class Singleton {
private static final Singleton INSTANCE = new Singleton();
private Singleton() {}
public static Singleton getInstance() {
return INSTANCE;
}
}
```
2. 懒汉式单例模式
在第一次使用时才创建单例对象,但线程不安全,需要加锁。
```java
public class Singleton {
private static Singleton INSTANCE;
private Singleton() {}
public static synchronized Singleton getInstance() {
if (INSTANCE == null) {
INSTANCE = new Singleton();
}
return INSTANCE;
}
}
```
3. 双重校验锁单例模式
既保证线程安全,又能在第一次使用时才创建单例对象,但需要注意volatile关键字的使用。
```java
public class Singleton {
private static volatile Singleton INSTANCE;
private Singleton() {}
public static Singleton getInstance() {
if (INSTANCE == null) {
synchronized (Singleton.class) {
if (INSTANCE == null) {
INSTANCE = new Singleton();
}
}
}
return INSTANCE;
}
}
```
4. 静态内部类单例模式
利用静态内部类的特性,在第一次使用时才创建单例对象,且线程安全,是最佳实践。
```java
public class Singleton {
private Singleton() {}
private static class SingletonHolder {
private static final Singleton INSTANCE = new Singleton();
}
public static Singleton getInstance() {
return SingletonHolder.INSTANCE;
}
}
```
相关推荐
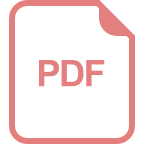














