python编写程序,爬取www.sdtbu.edu.cn网站上的所有新闻,每个新闻生成一个子文件夹,网页上的文本存放于一个文本文件,网页上的图片单独存放于该文件夹中
时间: 2024-03-30 14:40:25 浏览: 16
好的,这个问题需要编写一个爬虫程序来实现。我们可以使用Python的requests和BeautifulSoup库来获取网页内容和解析HTML。
首先,我们需要导入需要的库:
```python
import os
import requests
from bs4 import BeautifulSoup
```
然后,我们需要定义一个函数来获取网页内容,并使用BeautifulSoup来解析HTML:
```python
def get_html(url):
res = requests.get(url)
res.encoding = 'utf-8'
soup = BeautifulSoup(res.text, 'html.parser')
return soup
```
接下来,我们需要找到新闻列表的链接,并遍历每一个新闻链接。我们可以使用find_all函数来获取所有的新闻链接:
```python
url = 'http://www.sdtbu.edu.cn/'
soup = get_html(url)
news_list = soup.find_all('a', class_='news-title')
for news in news_list:
news_url = news['href']
news_title = news.text
print(news_url, news_title)
```
现在我们已经获取了每个新闻的链接和标题,接下来我们需要在本地创建一个子文件夹,并将新闻页面的内容和图片保存到该文件夹中。我们可以使用os库中的mkdir函数来创建文件夹,并使用requests库中的get函数来下载图片。
```python
for news in news_list:
news_url = news['href']
news_title = news.text
# 创建子文件夹
folder_name = news_title.replace('/', '-')
os.mkdir(folder_name)
# 获取新闻页面的内容和图片
news_soup = get_html(news_url)
content = news_soup.find('div', class_='content').text
with open(folder_name + '/content.txt', 'w', encoding='utf-8') as f:
f.write(content)
img_list = news_soup.find_all('img')
for i, img in enumerate(img_list):
img_url = img['src']
img_data = requests.get(img_url).content
with open(folder_name + '/img{}.jpg'.format(i), 'wb') as f:
f.write(img_data)
```
完整代码如下:
```python
import os
import requests
from bs4 import BeautifulSoup
def get_html(url):
res = requests.get(url)
res.encoding = 'utf-8'
soup = BeautifulSoup(res.text, 'html.parser')
return soup
url = 'http://www.sdtbu.edu.cn/'
soup = get_html(url)
news_list = soup.find_all('a', class_='news-title')
for news in news_list:
news_url = news['href']
news_title = news.text
# 创建子文件夹
folder_name = news_title.replace('/', '-')
os.mkdir(folder_name)
# 获取新闻页面的内容和图片
news_soup = get_html(news_url)
content = news_soup.find('div', class_='content').text
with open(folder_name + '/content.txt', 'w', encoding='utf-8') as f:
f.write(content)
img_list = news_soup.find_all('img')
for i, img in enumerate(img_list):
img_url = img['src']
img_data = requests.get(img_url).content
with open(folder_name + '/img{}.jpg'.format(i), 'wb') as f:
f.write(img_data)
```
相关推荐
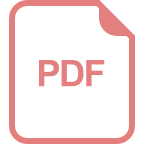














