Direct如何生成一张png图片、
时间: 2023-07-04 12:23:38 浏览: 135
DirectX本身并不直接支持生成PNG格式的图片,但是我们可以通过使用第三方库来实现将DirectX中的渲染结果保存为PNG格式的图片。以下是一个简单的流程:
1. 使用DirectX进行渲染,并将渲染结果存储在一个纹理中。
2. 将存储渲染结果的纹理复制到一个CPU可访问的内存中。
3. 使用第三方库(如libpng)将存储在内存中的渲染结果转换为PNG格式,并保存为文件。
具体实现步骤可以参考下面的代码示例:
```
// 创建渲染目标纹理
ID3D11Texture2D* renderTargetTexture;
D3D11_TEXTURE2D_DESC textureDesc;
ZeroMemory(&textureDesc, sizeof(textureDesc));
textureDesc.Width = screenWidth;
textureDesc.Height = screenHeight;
textureDesc.MipLevels = 1;
textureDesc.ArraySize = 1;
textureDesc.Format = DXGI_FORMAT_R8G8B8A8_UNORM;
textureDesc.SampleDesc.Count = 1;
textureDesc.Usage = D3D11_USAGE_DEFAULT;
textureDesc.BindFlags = D3D11_BIND_RENDER_TARGET | D3D11_BIND_SHADER_RESOURCE;
textureDesc.CPUAccessFlags = 0;
textureDesc.MiscFlags = 0;
device->CreateTexture2D(&textureDesc, NULL, &renderTargetTexture);
// 创建渲染目标视图
ID3D11RenderTargetView* renderTargetView;
D3D11_RENDER_TARGET_VIEW_DESC renderTargetViewDesc;
ZeroMemory(&renderTargetViewDesc, sizeof(renderTargetViewDesc));
renderTargetViewDesc.Format = textureDesc.Format;
renderTargetViewDesc.ViewDimension = D3D11_RTV_DIMENSION_TEXTURE2D;
renderTargetViewDesc.Texture2D.MipSlice = 0;
device->CreateRenderTargetView(renderTargetTexture, &renderTargetViewDesc, &renderTargetView);
// 设置渲染目标
deviceContext->OMSetRenderTargets(1, &renderTargetView, NULL);
// 渲染场景...
// 复制渲染结果到CPU可访问的内存中
D3D11_TEXTURE2D_DESC textureDesc;
renderTargetTexture->GetDesc(&textureDesc);
D3D11_TEXTURE2D_DESC copyTextureDesc;
ZeroMemory(©TextureDesc, sizeof(copyTextureDesc));
copyTextureDesc.Width = textureDesc.Width;
copyTextureDesc.Height = textureDesc.Height;
copyTextureDesc.MipLevels = 1;
copyTextureDesc.ArraySize = 1;
copyTextureDesc.Format = textureDesc.Format;
copyTextureDesc.SampleDesc.Count = 1;
copyTextureDesc.Usage = D3D11_USAGE_STAGING;
copyTextureDesc.CPUAccessFlags = D3D11_CPU_ACCESS_READ;
copyTextureDesc.MiscFlags = 0;
ID3D11Texture2D* copyTexture;
device->CreateTexture2D(©TextureDesc, NULL, ©Texture);
deviceContext->CopyResource(copyTexture, renderTargetTexture);
// 将渲染结果转换为PNG格式并保存为文件
BYTE* imageData = new BYTE[textureDesc.Width * textureDesc.Height * 4];
deviceContext->CopyResource(copyTexture, renderTargetTexture);
D3D11_MAPPED_SUBRESOURCE mappedResource;
deviceContext->Map(copyTexture, 0, D3D11_MAP_READ, 0, &mappedResource);
BYTE* imageData = new BYTE[textureDesc.Width * textureDesc.Height * 4];
memcpy(imageData, mappedResource.pData, textureDesc.Width * textureDesc.Height * 4);
deviceContext->Unmap(copyTexture, 0);
stbi_write_png("renderResult.png", textureDesc.Width, textureDesc.Height, 4, imageData, textureDesc.Width * 4);
delete[] imageData;
// 清理资源
renderTargetTexture->Release();
renderTargetView->Release();
copyTexture->Release();
```
这段代码中使用了第三方库stb_image_write来实现将内存中的图像数据保存为PNG格式文件。需要注意的是,stb_image_write库并不是官方的库,使用前需要自行下载并添加到项目中。
阅读全文
相关推荐
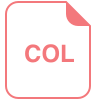
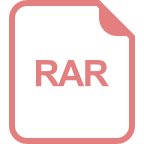
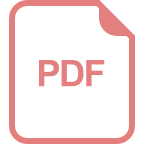
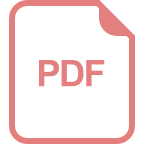
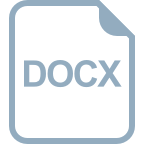
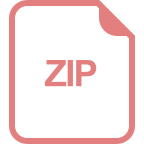
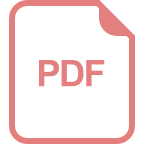
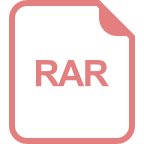
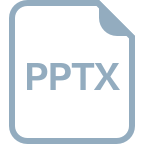
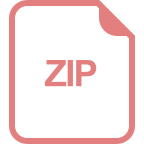
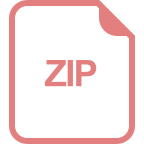
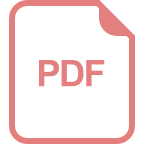
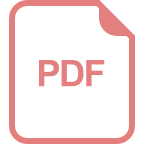