详细解释如下matlab中的自定义函数:function net = cnnsetup(net, x, y) assert(~isOctave() || compare_versions(OCTAVE_VERSION, '3.8.0', '>='), ['Octave 3.8.0 or greater is required for CNNs as there is a bug in convolution in previous versions. See http://savannah.gnu.org/bugs/?39314. Your version is ' myOctaveVersion]); inputmaps = 1; mapsize = size(squeeze(x(:, :, 1))); for l = 1 : numel(net.layers) % layer if strcmp(net.layers{l}.type, 's') mapsize = mapsize / net.layers{l}.scale; assert(all(floor(mapsize)==mapsize), ['Layer ' num2str(l) ' size must be integer. Actual: ' num2str(mapsize)]); for j = 1 : inputmaps net.layers{l}.b{j} = 0; end end if strcmp(net.layers{l}.type, 'c') mapsize = mapsize - net.layers{l}.kernelsize + 1; fan_out = net.layers{l}.outputmaps * net.layers{l}.kernelsize ^ 2; for j = 1 : net.layers{l}.outputmaps % output map fan_in = inputmaps * net.layers{l}.kernelsize ^ 2; for i = 1 : inputmaps % input map net.layers{l}.k{i}{j} = (rand(net.layers{l}.kernelsize) - 0.5) * 2 * sqrt(6 / (fan_in + fan_out)); net.layers{l}.dk_old{i}{j} = zeros(size(net.layers{l}.k{i}{j})); net.layers{l}.dk{i}{j} = net.layers{l}.dk_old{i}{j}; end net.layers{l}.b{j} = 0; end inputmaps = net.layers{l}.outputmaps; end end % 'onum' is the number of labels, that's why it is calculated using size(y, 1). If you have 20 labels so the output of the network will be 20 neurons. % 'fvnum' is the number of output neurons at the last layer, the layer just before the output layer. % 'ffb' is the biases of the output neurons. % 'ffW' is the weights between the last layer and the output neurons. Note that the last layer is fully connected to the output layer, that's why the size of the weights is (onum * fvnum) fvnum = prod(mapsize) * inputmaps; onum = size(y, 1); net.ffb = zeros(onum, 1); net.ffW = (rand(onum, fvnum) - 0.5) * 2 * sqrt(6 / (onum + fvnum)); end
时间: 2023-06-26 19:07:42 浏览: 47
这是一个用于设置卷积神经网络的自定义函数,函数名为“cnnsetup”,其输入参数为“net”、“x”和“y”,输出参数为“net”。该函数的目的是初始化神经网络的参数。
首先,在该函数中使用assert函数进行判断,如果运行该函数的Octave版本小于3.8.0,则会抛出异常,并给出一个链接以解决这个问题。
接下来,该函数会计算输入数据的通道数,并确定第一层卷积层的输出尺寸。然后,对于每一层,根据该层的类型和参数对输出尺寸进行更新,并初始化该层的权重和偏置。
对于卷积层,该函数会根据卷积核的大小、输入和输出通道数等参数初始化该层的权重。对于全连接层,该函数会计算全连接层的输入和输出尺寸,并初始化该层的权重和偏置。
最后,该函数返回初始化后的神经网络参数“net”。
相关问题
import pytest class test100: def test1(self): assert 1==1 if __name__=='_main_': pytest.main([__name__])
这段代码中,你定义了一个名为test100的类,并在其中定义了一个名为test1的方法。在test1方法中,你使用了pytest库的assert语句来判断1是否等于1。然后,你使用了一个条件判断语句,检查当前脚本是否作为主程序运行。如果是主程序运行,就调用pytest库的main函数来执行当前模块中的所有测试用例。
需要注意的是,正确的条件判断应该是`if __name__=='__main__':`,而不是`if __name__=='_main_':`。双下划线在两边都要有。此外,你需要将代码放在一个.py文件中才能运行。
def get_k_fold_data(k, i, X, y): assert k > 1 fold_size = X.shape[0] // k X_train, y_train = None, None for j in range(k): idx = slice(j * fold_size, (j + 1) * fold_size) X_part, y_part = X[idx,:], y[idx] if j == i: X_valid, y_valid = X_part, y_part elif X_train is None: X_train, y_train = X_part, y_part else: X_train = nd.concat(X_train, X_part, dim=0) y_train = nd.concat(y_train, y_part, dim=0) return X_train, y_train, X_valid, y_valid 对代码进行注释
# 定义一个函数,用于生成 k 折交叉验证数据集
# k: 折数
# i: 当前为第 i 折作为验证集
# X: 特征数据
# y: 标签数据
def get_k_fold_data(k, i, X, y):
# 断言 k 的值必须大于 1
assert k > 1
# 计算每一折数据集的大小
fold_size = X.shape[0] // k
# 初始化训练集和验证集的特征数据和标签数据
X_train, y_train = None, None
# 遍历每一折数据集
for j in range(k):
# 计算当前折数据集的索引范围
idx = slice(j * fold_size, (j + 1) * fold_size)
# 划分出当前折的特征数据和标签数据作为验证集
X_part, y_part = X[idx,:], y[idx]
if j == i:
# 如果当前折是验证集,则将其作为验证集
X_valid, y_valid = X_part, y_part
elif X_train is None:
# 如果当前训练集为空,则将当前折的特征数据和标签数据作为训练集
X_train, y_train = X_part, y_part
else:
# 如果当前训练集不为空,则在训练集的特征数据和标签数据后面拼接上当前折的特征数据和标签数据
X_train = nd.concat(X_train, X_part, dim=0)
y_train = nd.concat(y_train, y_part, dim=0)
# 返回训练集和验证集的特征数据和标签数据
return X_train, y_train, X_valid, y_valid
相关推荐
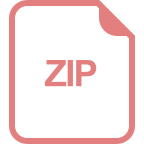
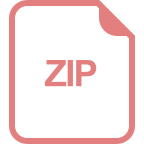
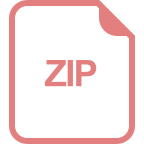













