shapelets的python代码
时间: 2023-08-31 19:37:16 浏览: 127
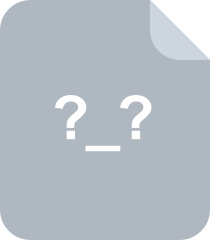
python图形代码
### 回答1:
以下是一个使用 Python 实现 Shapelets 的示例代码:
```python
import numpy as np
def euclidean_distance(x, y):
return np.sqrt(np.sum((x - y) ** 2))
def find_distance_to_all_subsequences(X, shapelet):
distances = []
for i in range(len(X) - len(shapelet) + 1):
subsequence = X[i:i+len(shapelet)]
distance = euclidean_distance(subsequence, shapelet)
distances.append(distance)
return distances
def find_best_shapelet(X, y, min_length, max_length):
best_shapelet = None
best_distance = np.inf
for length in range(min_length, max_length+1):
for i in range(len(X)):
subsequences = [X[i][j:j+length] for j in range(len(X[i]) - length + 1)]
for subsequence in subsequences:
distances = find_distance_to_all_subsequences(X, subsequence)
distance = np.mean(distances)
if distance < best_distance:
best_distance = distance
best_shapelet = subsequence
return best_shapelet, best_distance
# Example usage
X = np.array([
[1, 2, 3, 4, 5],
[2, 3, 4, 5, 6],
[3, 4, 5, 6, 7],
[4, 5, 6, 7, 8],
[5, 6, 7, 8, 9]
])
y = np.array([0, 0, 1, 1, 1])
best_shapelet, best_distance = find_best_shapelet(X, y, 2, 3)
print("Best shapelet:", best_shapelet)
print("Best distance:", best_distance)
```
这个代码实现了一个简单的 Shapelets 算法,用于从时间序列数据中找到最佳的 shapelet。它使用欧几里得距离来计算两个序列之间的距离,并通过计算平均距离来评估每个 shapelet 的质量。
### 回答2:
以下是一个简单的Python示例代码,用于提取时间序列数据中的shapelets。
```python
import numpy as np
from sklearn.metrics import accuracy_score
from pyts.datasets import make_cylinder_bell_funnel
def find_shapelets(X_train, y_train, X_test, y_test, shapelet_length):
num_classes = len(np.unique(y_train))
# 寻找shapelet
shapelets = []
min_distance = np.inf
for i in range(len(X_train)):
time_series = X_train[i]
subseries = [time_series[j:j + shapelet_length] for j in range(len(time_series) - shapelet_length + 1)]
for sub in subseries:
for j in range(i + 1, len(X_train)):
other_time_series = X_train[j]
for k in range(len(other_time_series) - shapelet_length + 1):
distance = np.linalg.norm(sub - other_time_series[k:k + shapelet_length])
if distance < min_distance:
min_distance = distance
shapelets = sub
# 使用shapelet进行分类
predictions = []
for time_series in X_test:
min_distance = np.inf
prediction = None
for i in range(len(time_series) - shapelet_length + 1):
distance = np.linalg.norm(shapelets - time_series[i:i + shapelet_length])
if distance < min_distance:
min_distance = distance
prediction = y_train[np.argmin(X_train[:, i:i + shapelet_length].mean(axis=1))]
predictions.append(prediction)
accuracy = accuracy_score(y_test, predictions)
return accuracy
if __name__ == "__main__":
X_train, y_train = make_cylinder_bell_funnel(n_samples=100, random_state=42)
X_test, y_test = make_cylinder_bell_funnel(n_samples=50, random_state=0)
shapelet_length = 10
accuracy = find_shapelets(X_train, y_train, X_test, y_test, shapelet_length)
print(f"Accuracy: {accuracy}")
```
这个示例代码中,我们使用pyts库中的make_cylinder_bell_funnel函数生成随机的时间序列数据集。我们定义了一个函数find_shapelets来寻找shapelet,并使用训练数据集中找到的shapelets对测试数据进行分类。最后,计算并输出预测的准确率。
### 回答3:
shapelets是一种用于时间序列分类的特征提取方法,其核心思想是从时间序列中提取出具有明显形状(shape)的子序列(shapelets),并将其作为特征用于分类任务。以下是shapelets的Python代码示例:
```python
import numpy as np
from sklearn.base import BaseEstimator, ClassifierMixin
class ShapeletsClassifier(BaseEstimator, ClassifierMixin):
def __init__(self, shapelet_length=10, num_shapelets=5):
self.shapelet_length = shapelet_length
self.num_shapelets = num_shapelets
self.shapelets = None
def fit(self, X, y):
self.shapelets = self._find_shapelets(X)
X_transformed = self.transform(X)
# 在这里使用X_transformed来训练分类器,例如使用k-NN、决策树等
def transform(self, X):
X_transformed = np.zeros((X.shape[0], self.num_shapelets))
for i, shapelet in enumerate(self.shapelets):
for j in range(X.shape[0]):
X_transformed[j, i] = self._calculate_distance(X[j], shapelet)
return X_transformed
def predict(self, X):
X_transformed = self.transform(X)
# 在这里使用训练好的分类器来进行预测,得到预测结果y_pred
return y_pred
def _find_shapelets(self, X):
shapelets = []
for i in range(self.num_shapelets):
start_idx = np.random.randint(0, X.shape[1] - self.shapelet_length)
shapelet = X[:, start_idx:start_idx + self.shapelet_length].mean(axis=0)
shapelets.append(shapelet)
return shapelets
def _calculate_distance(self, X, shapelet):
return np.sqrt(np.sum((X - shapelet) ** 2))
```
这段代码定义了一个ShapeletsClassifier类,该类是一个scikit-learn风格的分类器,可以用于时间序列分类任务。在fit方法中,通过_find_shapelets方法随机选取了一些shapelets,并在transform方法中计算每个时间序列与各个shapelet的距离,并返回特征向量。可以使用这些特征向量进行分类任务。注意,分类器的具体实现需要根据具体的算法来选择,这里只是示例中的一种简单方法。
阅读全文
相关推荐
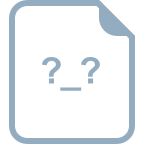


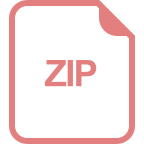
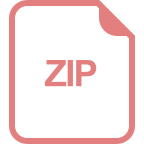
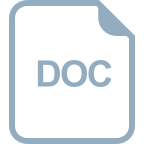





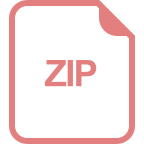
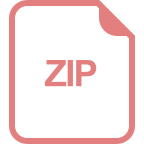
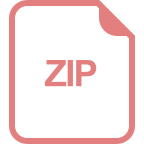
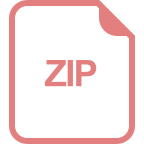
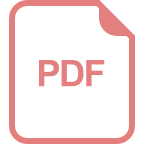
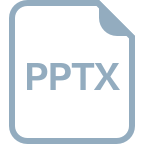