trilinear的python代码
时间: 2023-10-11 15:07:04 浏览: 213
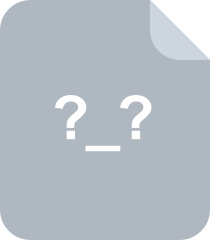
三角网格代码

以下是一个使用 NumPy 实现 trilinear 插值的 Python 代码示例:
```python
import numpy as np
def trilinear_interp(xyz, uvw, data):
"""
Trilinear interpolation of a 3D volume.
xyz: 3D point to be interpolated, in (x, y, z) format
uvw: normalized coordinates within the volume, in (u, v, w) format
data: 3D volume data
"""
# Get the integer coordinates of the 8 corner points
i, j, k = np.floor(xyz).astype(int)
# Get the fractional distances between the point and the corners
di, dj, dk = xyz - np.floor(xyz)
# Calculate the weights for each corner point
w000 = (1 - di) * (1 - dj) * (1 - dk)
w001 = (1 - di) * (1 - dj) * dk
w010 = (1 - di) * dj * (1 - dk)
w011 = (1 - di) * dj * dk
w100 = di * (1 - dj) * (1 - dk)
w101 = di * (1 - dj) * dk
w110 = di * dj * (1 - dk)
w111 = di * dj * dk
# Interpolate along the x-axis at y = j, z = k
c00 = w000 * data[i, j, k] + w100 * data[i+1, j, k]
c01 = w001 * data[i, j, k+1] + w101 * data[i+1, j, k+1]
c10 = w010 * data[i, j+1, k] + w110 * data[i+1, j+1, k]
c11 = w011 * data[i, j+1, k+1] + w111 * data[i+1, j+1, k+1]
c0 = (1 - dw) * c00 + dw * c01
c1 = (1 - dw) * c10 + dw * c11
# Interpolate along the y-axis at z = k
c = (1 - dv) * c0 + dv * c1
# Interpolate along the z-axis
result = (1 - du) * c + du * data[i, j, k+1]
return result
```
其中,`xyz` 是要进行插值的点的坐标,`uvw` 是该点在数据体中的归一化坐标,`data` 是一个三维的数据体。函数返回在该点处的插值结果。该函数假定数据体是规则的(即等间距采样),并且输入的归一化坐标是在数据体范围内的。
阅读全文
相关推荐
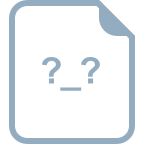
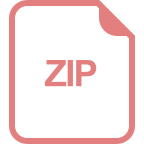



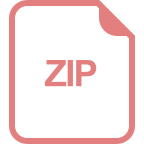
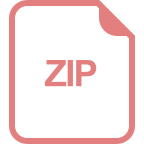
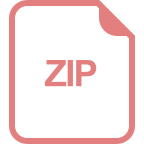
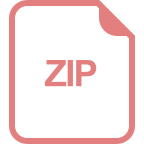
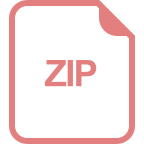