python三位数读取个十百多种方法
时间: 2024-09-18 15:02:16 浏览: 38
在Python中,读取一个三位数并将其分解为个位、十位和百位,有几种常见的方法可以实现:
1. **暴力拆分法**:将数字字符串转换为整数,然后通过除法和模运算获取每一位。例如:
```python
def split_number(num):
num_str = str(num)
last_digit = int(num_str[-1])
tenth_digit = int(num_str[-2]) if len(num_str) > 1 else 0
hundred_digit = int(num_str[0]) if len(num_str) >= 3 else 0
return hundred_digit, tenth_digit, last_digit
number = 123
hundred, tenth, last = split_number(number)
```
2. **列表解析式**:也可以使用列表解析式一次性完成这个任务,更简洁一些:
```python
number = 123
digits = [int(digit) for digit in str(number)]
hundred, tenth, last = digits[0], digits[1], digits[2] if len(digits) == 3 else (0, 0, digits[0])
```
3. **内置函数`divmod()`**:利用Python的内建函数`divmod()`可以同时得到商和余数,结合整除和取模操作:
```python
number = 123
hundred, remainder = divmod(number, 100)
tenth, last = divmod(remainder, 10), remainder % 10
```
阅读全文
相关推荐
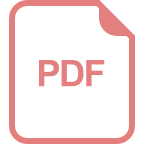
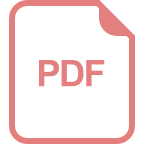
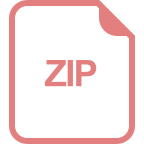















