写一个i.mx6 solox 的linux gpu 驱动代码
时间: 2024-02-11 21:04:16 浏览: 141
以下是一个简单的步骤,可以帮助您开始编写i.mx6 solox的linux gpu驱动程序:
1. 了解i.mx6 solox的GPU硬件架构和工作原理。
2. 阅读i.mx6 solox的GPU手册和文档,以便了解如何在Linux内核中启用GPU和配置GPU驱动程序。
3. 创建一个新的驱动程序或修改现有的驱动程序,以支持i.mx6 solox的GPU硬件。
4. 在Linux内核中注册GPU驱动程序,并确保正确的初始化和绑定GPU驱动程序。
5. 实现GPU的显示功能,并支持GPU的基本操作,如图形渲染、纹理映射等。
6. 可以实现高级的GPU操作,如3D渲染、硬件加速等。
7. 测试和调试GPU驱动程序,以确保其在i.mx6 solox硬件上正确工作。
以下是一个简单的i.mx6 solox的linux gpu驱动程序代码示例,供参考:
```c
#include <linux/module.h>
#include <linux/kernel.h>
#include <linux/init.h>
#include <linux/platform_device.h>
#include <linux/of.h>
#include <linux/of_device.h>
static int imx6solox_gpu_probe(struct platform_device *pdev)
{
struct device *dev = &pdev->dev;
struct resource *res;
void __iomem *regs;
int ret;
res = platform_get_resource(pdev, IORESOURCE_MEM, 0);
if (!res) {
dev_err(dev, "failed to get memory resource\n");
return -ENODEV;
}
regs = devm_ioremap_resource(dev, res);
if (IS_ERR(regs)) {
dev_err(dev, "failed to remap memory resource\n");
return PTR_ERR(regs);
}
/* TODO: initialize GPU hardware and register GPU device */
return 0;
}
static int imx6solox_gpu_remove(struct platform_device *pdev)
{
/* TODO: deinitialize GPU hardware and unregister GPU device */
return 0;
}
static const struct of_device_id imx6solox_gpu_match[] = {
{ .compatible = "fsl,imx6solox-gpu", },
{},
};
MODULE_DEVICE_TABLE(of, imx6solox_gpu_match);
static struct platform_driver imx6solox_gpu_driver = {
.driver = {
.name = "imx6solox-gpu",
.of_match_table = imx6solox_gpu_match,
},
.probe = imx6solox_gpu_probe,
.remove = imx6solox_gpu_remove,
};
module_platform_driver(imx6solox_gpu_driver);
MODULE_AUTHOR("Your Name");
MODULE_DESCRIPTION("i.mx6 solox GPU driver");
MODULE_LICENSE("GPL");
```
该驱动程序使用了Platform设备模型,使用了`devm_ioremap_resource()`函数将GPU的物理内存地址映射到虚拟地址空间,然后初始化GPU硬件并注册GPU设备。您可以在此基础上添加您所需要的GPU硬件操作,以实现完整的GPU驱动程序。
阅读全文
相关推荐
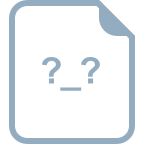
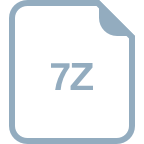
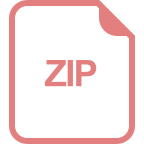
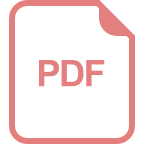
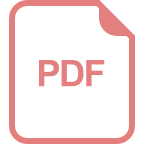
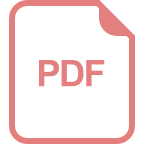
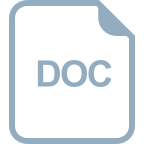
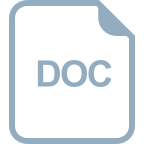
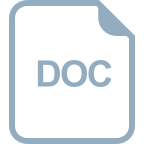
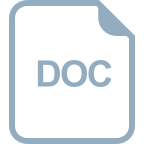
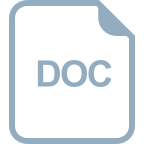
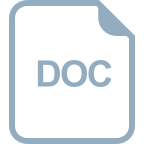
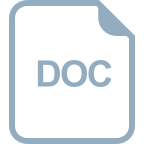
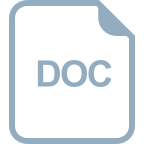
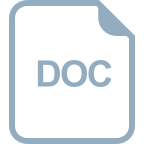
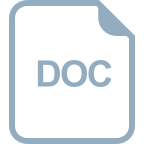
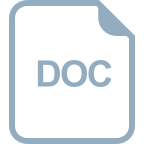