用C语言写一个通讯录排序的嵌套结构
时间: 2023-10-31 10:04:33 浏览: 112
以下是一个使用嵌套结构的通讯录排序程序,使用C语言编写:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_CONTACTS 100 // 最多联系人数
struct contact {
char name[50];
char phone[20];
};
int compare_contacts(const void *a, const void *b) {
struct contact *ca = (struct contact *)a;
struct contact *cb = (struct contact *)b;
return strcmp(ca->name, cb->name);
}
int main() {
int num_contacts;
struct contact contacts[MAX_CONTACTS];
printf("请输入联系人数目: ");
scanf("%d", &num_contacts);
getchar(); // 清除输入缓冲区中的回车符
// 输入联系人信息
for (int i = 0; i < num_contacts; i++) {
printf("请输入第%d个联系人姓名: ", i + 1);
fgets(contacts[i].name, sizeof(contacts[i].name), stdin);
contacts[i].name[strcspn(contacts[i].name, "\n")] = '\0'; // 去掉姓名字符串的换行符
printf("请输入第%d个联系人电话号码: ", i + 1);
fgets(contacts[i].phone, sizeof(contacts[i].phone), stdin);
contacts[i].phone[strcspn(contacts[i].phone, "\n")] = '\0'; // 去掉电话号码字符串的换行符
}
// 按姓名排序
qsort(contacts, num_contacts, sizeof(struct contact), compare_contacts);
// 输出排序后的联系人信息
printf("已排序的联系人列表:\n");
for (int i = 0; i < num_contacts; i++) {
printf("%s\t%s\n", contacts[i].name, contacts[i].phone);
}
return 0;
}
```
程序首先定义了一个结构体`contact`,其中包含了联系人的姓名和电话号码。然后定义了一个比较函数`compare_contacts`,用于比较两个联系人姓名的大小关系。接着在`main`函数中,程序先输入联系人数目,然后使用`fgets`函数逐个输入每个联系人的姓名和电话号码,并去掉字符串中的换行符。最后使用`qsort`函数按照姓名对联系人进行排序,然后输出排序后的联系人列表。
需要注意的是,在使用`fgets`函数输入字符串后,字符串末尾通常会包含一个换行符,因此需要使用`strcspn`函数去掉字符串中的换行符。
阅读全文
相关推荐
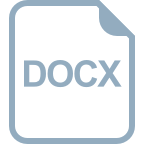
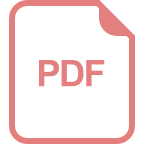
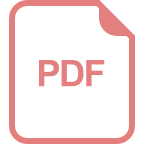











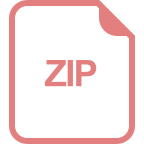
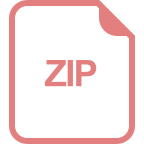
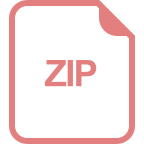
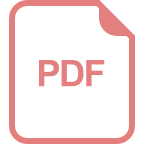