vue2中使用node中fs模块教程
时间: 2024-10-13 08:07:45 浏览: 117
在 Vue.js (版本2) 中,Node.js 的 `fs` 模块主要用于文件系统操作,如读取、写入、删除文件等。要在 Vue 组件中使用它,你需要在 Node 环境下编写服务端代码(通常用 Express 或其他框架),而不是直接在浏览器中。
以下是一个简单的步骤:
1. **引入 fs 模块**:
在你的 Node.js 服务器端代码(例如一个 `.js` 文件)里,首先导入 `fs` 模块:
```javascript
const fs = require('fs');
```
2. **读取文件**:
使用 `fs.readFile()` 或 `fs.promises.readFile()` 来读取文件内容,这在服务器启动时或需要预加载数据时很有用:
```javascript
const readFile = async (filePath) => {
try {
const data = await fs.promises.readFile(filePath, 'utf8'); // 以 utf8 编码读取
return data;
} catch (err) {
console.error(`Error reading file: ${err}`);
}
};
```
3. **写入文件**:
可以用 `fs.writeFile()` 或 `fs.promises.writeFile()` 来保存数据到文件:
```javascript
const writeFile = async (filePath, content) => {
try {
await fs.promises.writeFile(filePath, content, 'utf8');
console.log('File saved successfully');
} catch (err) {
console.error(`Error writing to file: ${err}`);
}
};
```
4. **Vue 组件中的调用**:
如果你有一个 Vue 面向服务端的组件,可以在 mounted 或生命周期钩子里调用上述函数,比如通过 Axios 发送 HTTP 请求到 Node 服务器来处理文件操作。
```javascript
export default {
data() {
return {
filePath: '/path/to/file',
};
},
created() {
this.readFile();
},
methods: {
readFile() {
this.$axios.post('/read-file', { filePath: this.filePath }).then(response => {
// response.data 包含文件内容
});
},
writeFile(newContent) {
this.$axios.post('/write-file', { filePath: this.filePath, content: newContent }).then(() => {
console.log('File written');
});
},
},
};
```
阅读全文
相关推荐
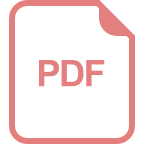
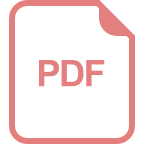
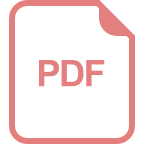


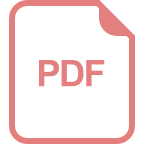
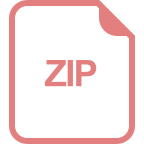
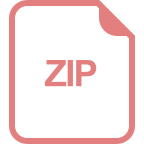
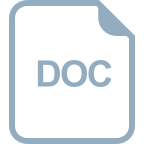
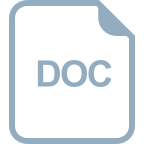
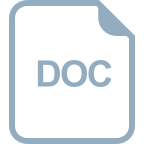
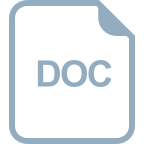
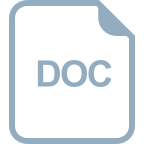
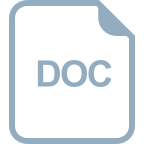
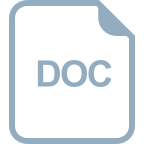


