购物管理系统c++代码
时间: 2024-12-24 16:10:34 浏览: 7
在创建购物管理系统的一个基本C++代码示例中,我们可能会涉及到几个关键组件,如用户界面、商品管理、订单处理等。这里提供一个简单的框架:
```cpp
#include <iostream>
#include <string>
#include <map>
// 商品类
class Product {
public:
std::string name;
double price;
// 构造函数
Product(const std::string& name, const double price) : name(name), price(price) {}
};
// 购物车类
class ShoppingCart {
private:
std::map<std::string, int> products; // 存放商品名及其数量
public:
void addItem(Product item, int quantity) {
if (products.find(item.name) != products.end()) {
products[item.name] += quantity;
} else {
products[item.name] = quantity;
}
}
void removeItem(Product item, int quantity) {
if (products.find(item.name) != products.end() && products[item.name] >= quantity) {
products[item.name] -= quantity;
} else {
std::cout << "商品不足!" << std::endl;
}
}
double getTotalCost() {
double total = 0;
for (const auto& pair : products) {
total += pair.first.price * pair.second;
}
return total;
}
};
int main() {
// 创建商品实例
Product apple("苹果", 5.0);
Product banana("香蕉", 3.0);
// 创建购物车
ShoppingCart cart;
// 添加物品到购物车
cart.addItem(apple, 2);
cart.addItem(banana, 1);
// 显示购物车总成本
std::cout << "购物车总成本: " << cart.getTotalCost() << "元" << std::endl;
return 0;
}
```
这个代码只是一个简化的版本,实际的购物管理系统会更复杂,可能包括数据库操作、库存管理、用户登录等功能。如果你需要详细了解如何设计和实现完整的购物管理系统,可以提问相关的具体问题,比如:
1. 如何设计数据库模型来存储商品信息?
2. 用户如何添加删除商品以及查看购物车内容?
3. 订单结算和支付功能怎么实现?
阅读全文
相关推荐





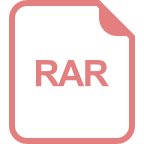

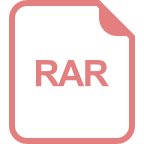









