定义一个结构体变量(包括年、月、日),输入一个日期,计算在本年中是第几天,注意闰年问题。
时间: 2024-12-23 08:21:11 浏览: 0
在 C 语言中,我们可以创建一个结构体来代表日期,包括年份、月份和天数。然后我们需要编写函数来计算给定日期在当年的第几天,考虑到闰年的规则。闰年是指能被4整除但不能被100整除的年份,或者是能被400整除的年份。
首先,定义一个结构体 `Date`:
```c
#include <stdio.h>
typedef struct {
int year;
int month;
int day;
} Date;
```
接下来,计算全年天数的函数(考虑闰年):
```c
int daysInYear(int year) {
if (year % 4 != 0 || (year % 100 == 0 && year % 400 != 0)) {
return 365; // 平年有365天
} else {
return 366; // 闰年有366天
}
}
int isLeapYear(int year) {
return (year % 4 == 0 && (year % 100 != 0 || year % 400 == 0));
}
```
现在,计算给定日期在当年的第几天:
```c
int calculateDay(Date date) {
int total_days = 0;
for (int i = 0; i < date.year; ++i) {
total_days += daysInYear(i);
}
if (isLeapYear(date.year)) {
total_days++;
}
total_days += (date.month - 1) * 31; // 简单地假设每个月都有31天
switch (date.month) {
case 2: total_days += isLeapYear(date.year) ? 29 : 28; break; // 考虑 February 的特殊天数
case 4: case 6: case 9: case 11: total_days += 30; break;
}
total_days += date.day;
return total_days;
}
```
这个函数 `calculateDay` 接收一个 `Date` 结构体实例,计算到该日期为止的所有天数,并返回结果。
阅读全文
相关推荐
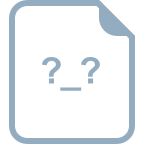
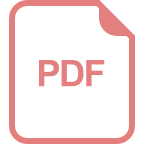
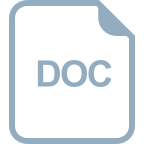















