编写一个查看手机属性与功能的程序,测试手机的各种属性和功能,用继承与封装
时间: 2024-09-14 17:09:54 浏览: 40
要编写一个查看手机属性与功能的程序,并使用继承与封装,我们可以定义一个基类来表示手机的基本属性和功能,然后通过继承机制让不同类型的手机(如智能手机、功能手机)展现其特有的属性和功能。封装是指将数据(属性)和代码(方法)包装在一起,对外部隐藏其细节,只提供公共接口进行交互。
以下是一个简化的示例,展示如何使用继承和封装来实现这个程序:
1. 定义一个抽象的基类`Phone`,它包含了手机的一些通用属性和方法,例如品牌、型号、内存大小、操作系统等。
```java
public abstract class Phone {
private String brand;
private String model;
private int memorySize;
private String operatingSystem;
public Phone(String brand, String model, int memorySize, String operatingSystem) {
this.brand = brand;
this.model = model;
this.memorySize = memorySize;
this.operatingSystem = operatingSystem;
}
// 封装的方法,用于获取手机信息
public String getBrand() {
return brand;
}
public String getModel() {
return model;
}
public int getMemorySize() {
return memorySize;
}
public String getOperatingSystem() {
return operatingSystem;
}
// 抽象方法,由子类实现
public abstract void checkFeatures();
}
```
2. 创建一个继承自`Phone`的子类`Smartphone`,它代表智能手机,并添加一些特有的属性和方法。
```java
public class Smartphone extends Phone {
private int cameraResolution;
private boolean hasTouchScreen;
public Smartphone(String brand, String model, int memorySize, String operatingSystem, int cameraResolution, boolean hasTouchScreen) {
super(brand, model, memorySize, operatingSystem);
this.cameraResolution = cameraResolution;
this.hasTouchScreen = hasTouchScreen;
}
@Override
public void checkFeatures() {
// 实现检查智能手机功能的方法
System.out.println("检查智能手机特性:");
System.out.println("品牌: " + getBrand());
System.out.println("型号: " + getModel());
System.out.println("内存大小: " + getMemorySize() + " GB");
System.out.println("操作系统: " + getOperatingSystem());
System.out.println("摄像头分辨率: " + cameraResolution + " MP");
System.out.println("是否有触屏: " + (hasTouchScreen ? "是" : "否"));
}
}
```
3. 实例化`Smartphone`类的对象,并调用其`checkFeatures()`方法来检查特性。
```java
public class PhoneTest {
public static void main(String[] args) {
Smartphone smartphone = new Smartphone("Xiaomi", "Mi 11", 8, "MIUI", 108, true);
smartphone.checkFeatures();
}
}
```
以上代码创建了一个简单的程序框架,用于查看和测试手机的属性和功能。当然,实际的手机测试程序可能会更加复杂,包含更多的属性、方法和功能测试。
阅读全文
相关推荐
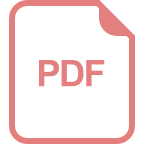
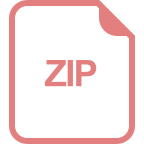
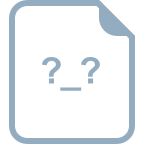
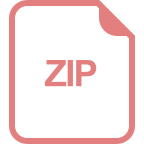
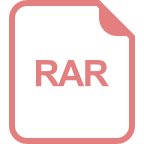
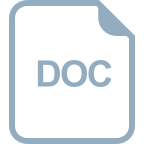
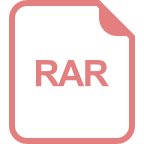
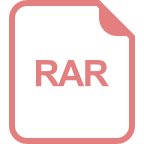
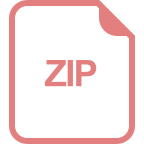
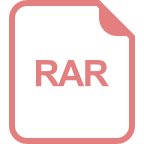
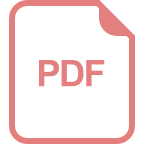
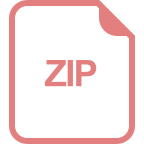
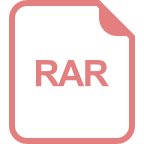
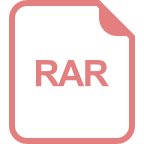
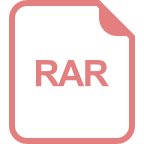
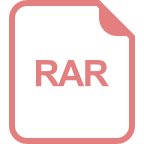
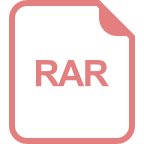
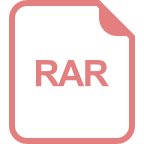
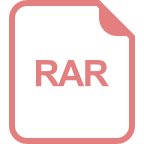