模拟退火算法c++模板
时间: 2024-09-10 16:01:17 浏览: 53
模拟退火算法(Simulated Annealing, SA)是一种通用概率算法,用来在一个大的搜寻空间内寻找足够好的解。它是由S. Kirkpatrick, C. D. Gelatt 和M. P. Vecchi 在1983年提出的。该算法受到物理学中固体物质退火过程的启发,其思想是高温时物质内部粒子处于高能态,随着温度慢慢降低,粒子逐渐趋向于能量较低的稳定态,这个过程就模拟了问题的求解过程。
在C++中,我们可以编写一个模拟退火算法的模板类,这个模板类可以接受不同问题的具体实现。下面是一个简化的模拟退火算法的C++模板类结构:
```cpp
#include <iostream>
#include <cmath>
#include <ctime>
#include <cstdlib>
template<typename T>
class SimulatedAnnealing {
public:
T currentSolution; // 当前解
T bestSolution; // 最佳解
double T_start; // 初始温度
double T_end; // 终止温度
double alpha; // 温度衰减系数
int max_iter; // 每个温度下的最大迭代次数
// 构造函数,初始化模拟退火算法参数
SimulatedAnnealing(T startSolution, double T_start, double T_end, double alpha, int max_iter)
: currentSolution(startSolution), bestSolution(startSolution), T_start(T_start), T_end(T_end), alpha(alpha), max_iter(max_iter) {}
// 开始算法
void start() {
double T = T_start;
while (T > T_end) {
for (int i = 0; i < max_iter; ++i) {
T = T * alpha;
T = std::max(T, T_end); // 防止温度过低
TSolution newSolution = generateNewSolution(currentSolution);
double cost = costFunction(newSolution);
double currentCost = costFunction(currentSolution);
if (acceptanceProbability(cost, currentCost, T) > (double)rand() / (RAND_MAX)) {
currentSolution = newSolution;
if (cost < costFunction(bestSolution)) {
bestSolution = newSolution;
}
}
}
}
}
// 生成新的解决方案
virtual T generateNewSolution(const T& currentSolution) = 0;
// 计算成本函数
virtual double costFunction(const T& solution) = 0;
// 接受概率函数
double acceptanceProbability(double cost, double currentCost, double T) {
return std::exp(-(cost - currentCost) / T);
}
};
// 示例使用
class TravelingSalesmanProblem {
public:
// 假设这里有一些与旅行商问题相关的数据和方法
};
int main() {
TravelingSalesmanProblem tsp;
SimulatedAnnealing<TravelingSalesmanProblem> sa(tsp, 1000.0, 1.0, 0.99, 100);
sa.start();
// 输出最佳解
std::cout << "Best solution cost: " << costFunction(sa.bestSolution) << std::endl;
return 0;
}
```
注意:上述代码是一个非常简化的模板示例,实际应用中需要根据具体问题定义`generateNewSolution`和`costFunction`方法。
阅读全文
相关推荐
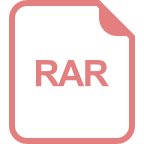
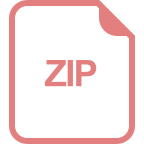
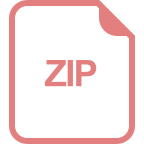
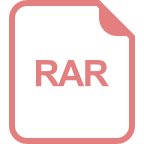
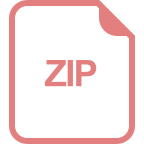
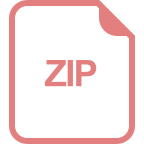
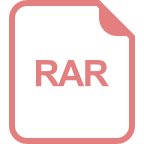
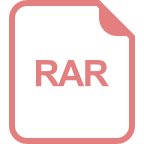
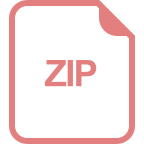
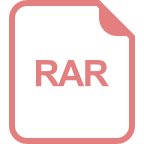
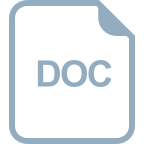
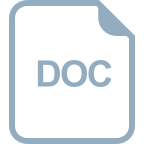
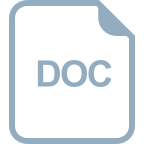
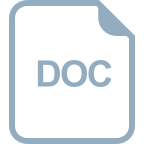
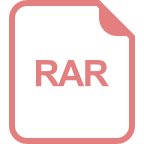
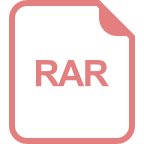
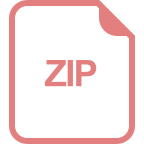
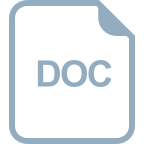
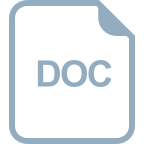