html php 带跨域上传多张图片的代码
时间: 2024-10-29 20:12:12 浏览: 24
HTML、PHP和处理跨域文件上传通常涉及到前端发送请求到后端服务器,这里简述一种常见的流程。首先,你需要在前端创建一个HTML表单让用户选择图片,并使用`FormData`对象来收集文件数据。然后,在PHP后端,需要设置允许跨域请求。
**前端(HTML & JavaScript)部分:**
```html
<!DOCTYPE html>
<html lang="en">
<head>
<script src="https://cdn.jsdelivr.net/npm/@axios/axios@0.27.2"></script>
</head>
<body>
<form id="uploadForm">
<input type="file" multiple accept="image/*" name="images[]">
<button type="submit">Upload</button>
</form>
<script>
document.getElementById('uploadForm').addEventListener('submit', async (e) => {
e.preventDefault();
const formData = new FormData(e.target);
try {
const response = await axios.post('/upload', formData, {
headers: {
'Content-Type': 'multipart/form-data',
'X-Requested-With': 'XMLHttpRequest'
},
withCredentials: true // 如果有跨域需求,添加此行
});
console.log(response.data); // 处理服务器返回的数据
} catch (error) {
console.error(error);
}
});
</script>
</body>
</html>
```
**后端(PHP)部分:**
```php
<?php
header("Access-Control-Allow-Origin: *"); // 允许跨域请求
header("Access-Control-Allow-Methods: POST");
header("Access-Control-Allow-Headers: X-Requested-With");
// 检查POST数据并保存图片
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
$target_dir = "uploads/";
$target_file = $target_dir . basename($_FILES["images"]["name"][$i]);
if(move_uploaded_file($_FILES["images"]["tmp_name"][$i], $target_file)) {
echo json_encode(array("status" => "success", "filename" => $target_file));
} else {
echo json_encode(array("status" => "error", "message" => "Failed to upload"));
}
}
?>
```
**注意事项:**
1. PHP脚本应该放在支持接收文件上传的Web服务器环境中(如Apache或Nginx)。
2. 跨域请求的安全设置需谨慎,尽量限制允许的源。
3.
阅读全文
相关推荐
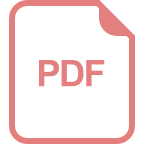
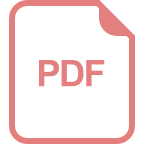
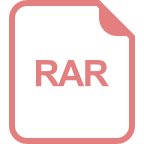


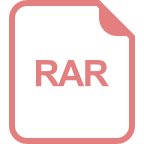
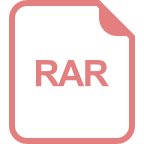
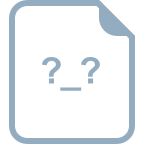
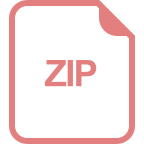
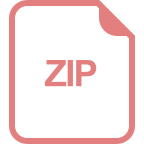
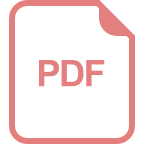
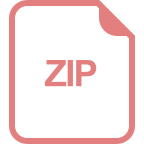
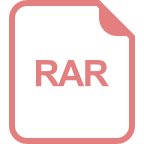
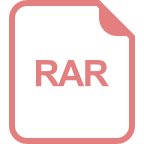
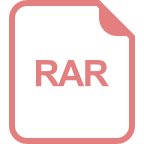
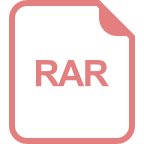
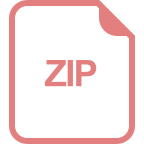
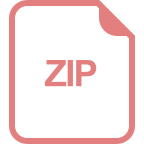
