如何用Java实现链式栈的数据结构?
时间: 2024-10-22 22:18:04 浏览: 10
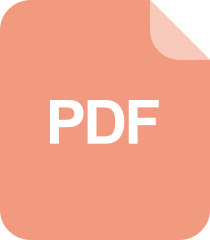
Java栈之链式栈存储结构的实现代码
在Java中,我们可以使用自定义类和引用来实现链式栈(也称为单向链表的栈)。下面是一个简单的例子:
首先,定义一个Node类作为栈节点,包含元素值和指向下一个节点的引用:
```java
class Node {
int data;
Node next;
public Node(int data) {
this.data = data;
this.next = null;
}
}
```
然后,创建一个Stack类,包含一个指向栈顶的Node引用:
```java
public class Stack {
private Node top;
// 添加元素到栈顶
public void push(int value) {
Node newNode = new Node(value);
if (top != null) {
newNode.next = top;
} else {
top = newNode;
}
}
// 删除并返回栈顶元素
public int pop() {
if (isEmpty()) {
throw new IllegalStateException("Stack is empty");
}
int value = top.data;
top = top.next;
return value;
}
// 检查栈是否为空
public boolean isEmpty() {
return top == null;
}
// 返回栈顶元素但不删除它
public int peek() {
if (isEmpty()) {
throw new IllegalStateException("Stack is empty");
}
return top.data;
}
}
```
现在你可以通过实例化Stack类来使用这个链式栈了。这就是链式栈的基本实现。
阅读全文
相关推荐
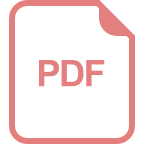
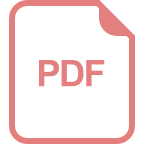
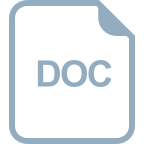
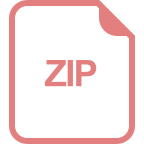
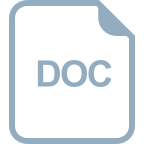
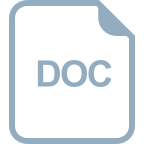




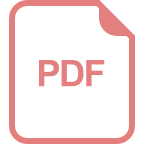
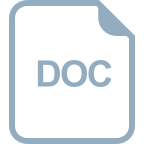
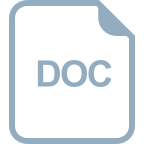
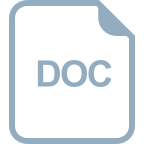
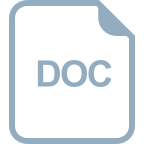



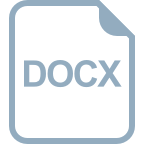