优化上述代码,使其能爬取目标excel文件中的url连接至对应的文件夹
时间: 2024-10-08 17:18:17 浏览: 16
如果你想优化这段代码,让它能够从Excel文件中读取URL并将其下载到特定的文件夹,你需要使用一些额外的库,比如`pandas`处理Excel文件,以及`os`模块来管理目录和文件操作。以下是一个简单的示例:
```python
import pandas as pd
from os import mkdir, path
# 读取Excel文件
def read_excel(url):
try:
df = pd.read_excel(url)
except Exception as e:
print(f"Error reading Excel file: {e}")
return None
else:
return df
# 下载文件到指定文件夹
def download_file(df, folder_path, url_column_name):
if not path.exists(folder_path):
mkdir(folder_path)
for index, row in df.iterrows():
url = row[url_column_name]
if isinstance(url, str) and url:
filename = path.join(folder_path, path.basename(url))
try:
response = requests.get(url, allow_redirects=True)
with open(filename, 'wb') as f:
f.write(response.content)
print(f"Downloaded '{filename}'")
except Exception as e:
print(f"Failed to download '{url}': {e}")
# 示例使用
file_url = "path_to_your_excel.xlsx"
folder_path = "target_folder"
url_column = "URL_Column_Name"
df = read_excel(file_url)
if df is not None:
download_file(df, folder_path, url_column)
```
这段代码首先读取Excel文件,然后遍历其中的一列(假设名为“URL_Column_Name”),对于每个有效URL,它会在指定的文件夹中创建一个子目录(如果不存在的话)并下载文件。请注意,这里使用了`requests`库来发送HTTP请求,所以需要先安装它(`pip install requests`)。
阅读全文
相关推荐
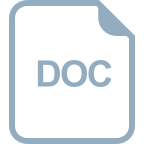
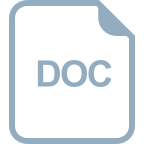
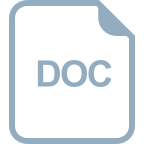
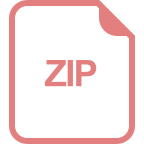
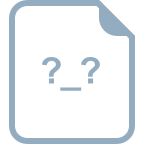
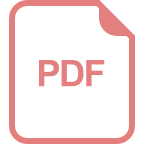
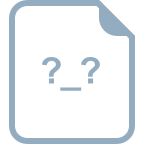
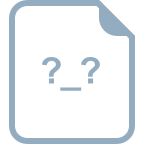
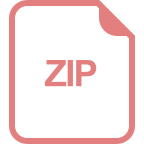
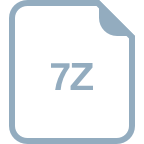
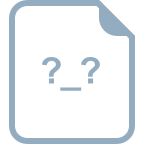