编写一个能计算收益的程序
时间: 2024-10-01 11:06:49 浏览: 31
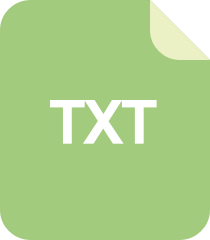
编写程序,计算理财收益中复利与单利的差额.txt
编写一个计算收益的程序通常涉及以下几个步骤,这里以Python为例,假设有一个简单的股票投资案例:
```python
class Stock:
def __init__(self, initial_price, quantity, final_price):
self.initial_price = initial_price
self.quantity = quantity
self.final_price = final_price
def calculate_profit(self):
profit = (self.final_price - self.initial_price) * self.quantity
return profit
# 创建实例并计算收益
stock = Stock(50, 100, 60)
profit = stock.calculate_profit()
print(f"总投资 {stock.initial_price * stock.quantity} 元,最终收益为 {profit} 元.")
阅读全文
相关推荐
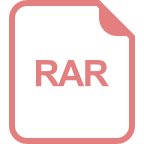
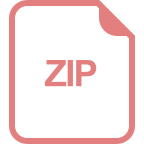
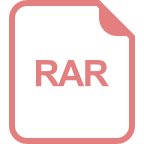
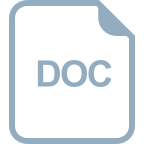













