if args.lr_decay: # True if args.lr_decay_interval and args.lr_step_decay_epochs: raise ValueError('lr_decay_interval and lr_step_decay_epochs are mutually exclusive!') if args.lr_step_decay_epochs: decay_epoch_list = [int(ep.strip()) for ep in args.lr_step_decay_epochs.split(',')] decay_rate_list = [float(rt.strip()) for rt in args.lr_step_decay_rates.split(',')]
时间: 2023-11-04 16:05:51 浏览: 196
这段代码首先判断`args.lr_decay`是否为真(True)。如果为真,则继续执行下面的逻辑。
接下来,代码会进一步判断`args.lr_decay_interval`和`args.lr_step_decay_epochs`是否同时存在。如果它们同时存在,会抛出一个`ValueError`异常,提示`lr_decay_interval`和`lr_step_decay_epochs`是互斥的选项,不能同时进行设置。
如果`args.lr_step_decay_epochs`存在,代码会进一步执行下面的逻辑。首先,将`args.lr_step_decay_epochs`按逗号(`,`)分隔成一个列表,并将每个元素转换为整数类型。这个列表包含了每个衰减阶段的时期(epoch)。接着,将`args.lr_step_decay_rates`按逗号分隔成另一个列表,并将每个元素转换为浮点数类型。这个列表包含了每个衰减阶段的衰减率(decay rate)。
这段代码的目的是根据用户提供的参数设置,生成衰减阶段(epoch)列表和对应的衰减率列表,以便在训练过程中使用。具体的用途可能是在指定的时期应用不同的学习率衰减策略来优化模型的训练效果。
相关问题
if args.optim == 'adam': optimizer = optim.Adam(model.parameters(), lr=args.lr_init, weight_decay=args.weight_decay) elif args.optim == 'sgd': optimizer = optim.SGD(model.parameters(), lr=args.lr_init, momentum=args.momentum, weight_decay=args.weight_decay) elif args.optim == 'adamw': optimizer = optim.AdamW(model.parameters(), lr = args.lr_init, weight_decay=args.weight_decay) elif args.optim == 'adam_lars': optimizer = optim.Adam(model.parameters(), lr = args.lr_init, weight_decay=args.weight_decay) optimizer = LARC(optimizer=optimizer, eps=1e-8, trust_coefficient=0.001) elif args.optim == 'sgd_lars': optimizer = optim.SGD(model.parameters(), lr=args.lr_init, momentum=args.momentum, weight_decay=args.weight_decay) optimizer = LARC(optimizer=optimizer, eps=1e-8, trust_coefficient=0.001) elif args.optim == 'adamw_lars': optimizer = optim.AdamW(model.parameters(), lr = args.lr_init, weight_decay=args.weight_decay) optimizer = LARC(optimizer=optimizer, eps=1e-8, trust_coefficient=0.001)
这段代码是用于选择优化器的,根据 `args.optim` 的不同值,选择使用不同的优化器进行模型参数的更新。目前支持的优化器包括 Adam、SGD、AdamW,以及带有 LARS(Layer-wise Adaptive Rate Scaling)优化器的 Adam、SGD、AdamW。其中,Adam、SGD、AdamW 是常用的优化器,LARS 是一种针对深度神经网络的优化方法,它针对每一层的学习率做出不同的调整,以加速训练过程并提高模型性能。在代码中,`model.parameters()` 表示要对模型的所有参数进行优化。
assert(args.solver in ['adam', 'sgd']) print('=> setting {} solver'.format(args.solver)) param_groups = [{'params': model.module.bias_parameters(), 'weight_decay': args.bias_decay}, {'params': model.module.weight_parameters(), 'weight_decay': args.weight_decay}] if args.solver == 'adam': optimizer = torch.optim.Adam(param_groups, args.lr, betas=(args.momentum, args.beta)) elif args.solver == 'sgd': optimizer = torch.optim.SGD(param_groups, args.lr, momentum=args.momentum)
这段代码用于选择并配置优化器(optimizer)来优化模型的参数。
首先,使用`assert`语句来确保`args.solver`的值是`'adam'`或`'sgd'`,如果不是,程序会抛出一个AssertionError。
接下来,根据`args.solver`的值选择相应的优化器,并打印出所选择的优化器。代码块中的if-elif条件语句用于根据`solver`的值来选择优化器,分别是Adam和SGD。
然后,根据模型的参数进行分组,并为每个参数组设置权重衰减(weight_decay)。这里使用了`model.module.bias_parameters()`和`model.module.weight_parameters()`来获取模型中的偏置参数和权重参数。
最后,根据选择的优化器和参数组,创建一个优化器对象,并传入学习率(args.lr),以及其他特定于优化器的参数(如Adam的betas或SGD的momentum)。
通过这段代码,可以根据命令行参数(args)选择并配置不同的优化器来训练模型。
阅读全文
相关推荐
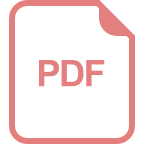
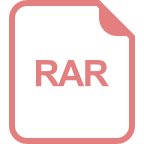
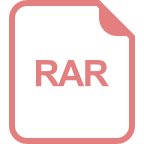



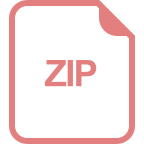
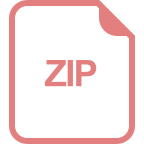
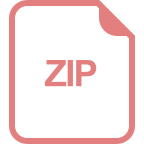
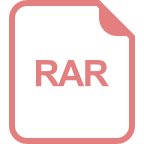
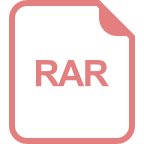
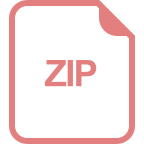
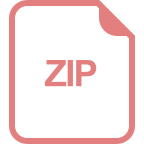


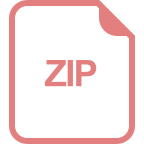