unity video capture
时间: 2023-09-22 14:13:07 浏览: 173
在Unity中进行视频捕捉可以使用Unity的VideoCapture API。以下是一个简单的示例代码,用于在Unity中进行视频捕捉:
```csharp
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.Video;
public class VideoCaptureExample : MonoBehaviour
{
public RawImage previewImage;
public VideoPlayer videoPlayer;
private VideoCapture videoCapture;
private bool isRecording = false;
void Start()
{
videoCapture = new VideoCapture();
previewImage.texture = videoCapture.previewTexture;
}
void Update()
{
if (Input.GetKeyDown(KeyCode.R))
{
if (!isRecording)
{
StartRecording();
}
else
{
StopRecording();
}
}
}
void StartRecording()
{
videoCapture.StartRecordingAsync(
"VideoClip",
OnStartedRecording,
OnFinishedRecording
);
}
void StopRecording()
{
videoCapture.StopRecordingAsync(OnStoppedRecording);
}
void OnStartedRecording(VideoCapture.VideoCaptureResult result)
{
if (result.success)
{
Debug.Log("Started recording");
isRecording = true;
}
else
{
Debug.LogError("Failed to start recording");
}
}
void OnFinishedRecording(VideoCapture.VideoCaptureResult result)
{
if (result.success)
{
Debug.Log("Finished recording");
isRecording = false;
// Play the recorded video
videoPlayer.url = result.outputFilePath;
videoPlayer.Play();
}
else
{
Debug.LogError("Failed to finish recording");
}
}
void OnStoppedRecording(VideoCapture.VideoCaptureResult result)
{
if (result.success)
{
Debug.Log("Stopped recording");
isRecording = false;
}
else
{
Debug.LogError("Failed to stop recording");
}
}
}
```
在这个示例中,我们首先创建了一个VideoCapture实例,并将预览纹理设置为RawImage组件的纹理。然后,我们在Update方法中检查是否按下了'R'键,以开始或停止录制。
当开始录制时,我们调用`StartRecordingAsync()`方法来开始录制视频。并通过回调函数`OnStartedRecording`来处理开始录制的结果。
当停止录制时,我们调用`StopRecordingAsync()`方法来停止录制。并通过回调函数`OnStoppedRecording`来处理停止录制的结果。
在完成录制后,我们可以使用VideoPlayer组件来播放已录制的视频。
请注意,VideoCapture API在不同平台上的支持可能有所不同。您可以参考Unity的文档以了解更多详细信息。
阅读全文
相关推荐
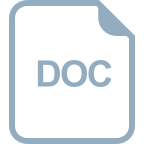
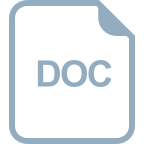
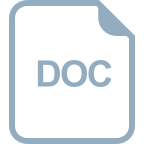
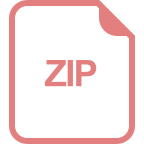


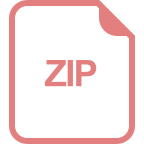
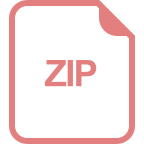


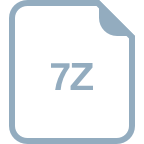
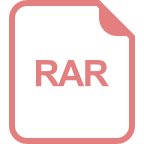
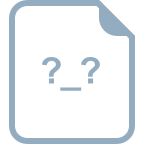
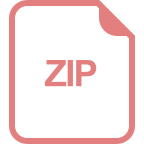
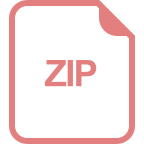