Spring Security Crypto支持SM2/3/4加密吗?如果支持,请给出代码示例
时间: 2024-01-15 12:03:34 浏览: 86
是的,Spring Security Crypto支持SM2/3/4加密,使用Bouncy Castle实现。下面是一个简单的示例代码:
```java
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import org.springframework.security.crypto.codec.Hex;
import java.nio.charset.StandardCharsets;
import java.security.Security;
public class SMExample {
public static void main(String[] args) {
// 添加Bouncy Castle算法提供者
Security.addProvider(new org.bouncycastle.jce.provider.BouncyCastleProvider());
// SM4加密/解密示例
byte[] keyBytes = Hex.decode("0123456789abcdeffedcba9876543210");
SM4Engine sm4 = new SM4Engine();
sm4.init(true, new KeyParameter(keyBytes));
byte[] input = "Hello, SM4!".getBytes(StandardCharsets.UTF_8);
byte[] output = new byte[sm4.getOutputSize(input.length)];
int len = sm4.processBytes(input, 0, input.length, output, 0);
sm4.doFinal(output, len);
System.out.println("SM4 encrypt result: " + Hex.encode(output));
sm4.init(false, new KeyParameter(keyBytes));
byte[] plaintext = new byte[sm4.getOutputSize(output.length)];
len = sm4.processBytes(output, 0, output.length, plaintext, 0);
sm4.doFinal(plaintext, len);
System.out.println("SM4 decrypt result: " + new String(plaintext, StandardCharsets.UTF_8));
// SM2加密/解密示例
SM2Engine sm2 = new SM2Engine();
ECPrivateKeyParameters privateKey = new ECPrivateKeyParameters(new BigInteger("123456"), sm2.getParameters());
ECPublicKeyParameters publicKey = new ECPublicKeyParameters(sm2.getParameters().getG().multiply(new BigInteger("123456")), sm2.getParameters());
sm2.init(true, new ParametersWithRandom(publicKey, new SecureRandom()));
byte[] data = "Hello, SM2!".getBytes(StandardCharsets.UTF_8);
byte[] encryptedData = sm2.processBlock(data, 0, data.length);
System.out.println("SM2 encrypt result: " + Hex.encode(encryptedData));
sm2.init(false, privateKey);
byte[] decryptedData = sm2.processBlock(encryptedData, 0, encryptedData.length);
System.out.println("SM2 decrypt result: " + new String(decryptedData, StandardCharsets.UTF_8));
}
}
```
需要注意的是,SM4和SM3算法不需要密钥对,而SM2算法需要密钥对。在示例代码中,我们使用Bouncy Castle提供的SM2算法实现了密钥对的生成,并使用SM2Engine进行加密和解密。
阅读全文
相关推荐








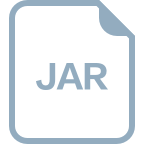




