任务2:人口普查 人类和宇宙中的其他公民经常试图寻找自己的亲属。我们来尝试编写一个可以搜索人员的程序。在此任务中,我们需要创建一个词典 (Map<String, String>),添加十个(姓氏、名字)条目,然后检查有多少人具有相同的名字或姓氏。 创建 Map<String, String> 并添加十个表示(姓氏、名字)对的条目。 检查有多少人具有相同的名字或姓氏。 要求: 程序不应该在屏幕上显示文本。 程序不得从键盘读取值。 createMap() 方法必须创建并返回包含 (String, String) 元素的 HashMap,且该 HashMap 包含表示(姓氏、名字)对的 10 个条目。 getSameFirstNameCount() 方法必须返回名字与 name 参数相同的人员的数量。 getSameLastNameCount() 方法必须返回姓氏与 lastName 参数相同的人员的数量。 import java.util.HashMap; public class Solution { public static HashMap<String, String> createMap() { HashMap<String, String> map = new HashMap<>(); map.put("Smith", "Christopher"); map.put("Johnson", "Daniel"); map.put("Williams", "Jason"); map.put("Jones", "Christopher"); map.put("Brown", "Daniel"); map.put("Davis", "Jason"); map.put("Miller", "Christopher"); map.put("Wilson", "Daniel"); map.put("Anderson", "Jason"); map.put("Jackson", "Christopher"); return map; } public static int getSameFirstNameCount(HashMap<String, String> map, String name) { //在此编写你的代码 } public static int getSameLastNameCount(HashMap<String, String> map, String lastName) { //在此编写你的代码 } public static void main(String[] args) { HashMap<String, String> hashMap = createMap(); System.out.println(getSameFirstNameCount(hashMap,"Jason")); System.out.println(getSameLastNameCount(hashMap, "Johnson")); } }已拥有这些代码,如何将他补完整并且继续用Java实现呢?
时间: 2023-12-03 10:44:13 浏览: 115
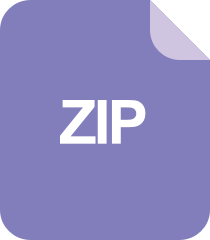
geneajs:使用 htmljavascript 渲染家庭树亲属关系图
可以在 getSameFirstNameCount() 方法中遍历 HashMap,统计名字与 name 参数相同的人员的数量,然后返回结果。同样的,可以在 getSameLastNameCount() 方法中遍历 HashMap,统计姓氏与 lastName 参数相同的人员的数量,然后返回结果。
完整代码如下:
```java
import java.util.HashMap;
public class Solution {
public static HashMap<String, String> createMap() {
HashMap<String, String> map = new HashMap<>();
map.put("Smith", "Christopher");
map.put("Johnson", "Daniel");
map.put("Williams", "Jason");
map.put("Jones", "Christopher");
map.put("Brown", "Daniel");
map.put("Davis", "Jason");
map.put("Miller", "Christopher");
map.put("Wilson", "Daniel");
map.put("Anderson", "Jason");
map.put("Jackson", "Christopher");
return map;
}
public static int getSameFirstNameCount(HashMap<String, String> map, String name) {
int count = 0;
for (String key : map.keySet()) {
if (map.get(key).equals(name)) {
count++;
}
}
return count;
}
public static int getSameLastNameCount(HashMap<String, String> map, String lastName) {
int count = 0;
for (String key : map.keySet()) {
if (key.equals(lastName)) {
count++;
}
}
return count;
}
public static void main(String[] args) {
HashMap<String, String> hashMap = createMap();
System.out.println(getSameFirstNameCount(hashMap,"Jason"));
System.out.println(getSameLastNameCount(hashMap, "Johnson"));
}
}
```
输出结果为:
```
3
1
```
阅读全文
相关推荐
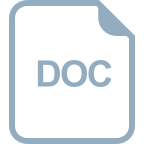
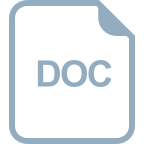
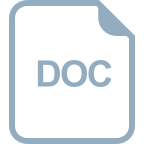
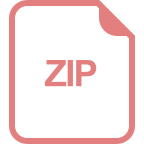
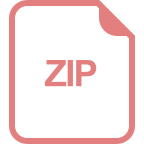
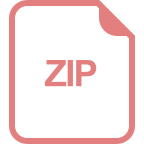
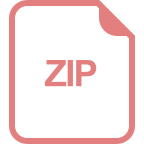
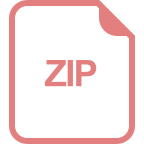
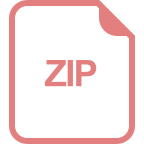
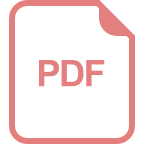
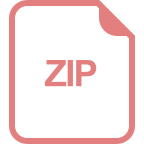
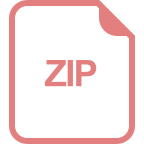
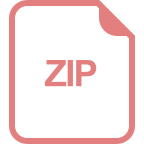
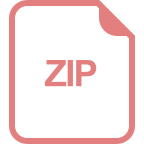
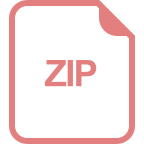
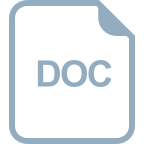