python 二维码识别的所有办法
时间: 2023-07-31 22:11:27 浏览: 57
除了使用 ZBar 库,还有其他方法可以在 Python 中识别二维码。以下是一些常用的方法:
1. pyzbar
pyzbar 是一个 Python 库,可以用于识别和解码常见的一维和二维条码。它基于 ZBar 库,并提供了更易于使用的接口。
示例代码:
```python
from pyzbar.pyzbar import decode
from PIL import Image
# 读取图像
img = Image.open('qrcode.png')
# 识别二维码
results = decode(img)
# 遍历识别结果
for result in results:
# 打印二维码类型和内容
print("Type:", result.type, "| Content:", result.data.decode("utf-8"))
```
2. OpenCV
OpenCV 是一个计算机视觉库,可以用于图像处理和分析。它可以用于检测和识别二维码。
示例代码:
```python
import cv2
import numpy as np
# 读取图像
img = cv2.imread('qrcode.png')
# 转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 使用 cv2.QRCodeDetector 检测二维码
detector = cv2.QRCodeDetector()
data, bbox, _ = detector.detectAndDecode(gray)
# 如果检测到二维码,打印内容
if bbox is not None:
print("Content:", data)
```
3. zxing
zxing 是一个开源的条码和二维码处理库,支持多种编程语言。在 Python 中,可以使用 pyzxing 库来调用 zxing。
示例代码:
```python
from pyzxing import BarCodeReader
# 创建 BarCodeReader 对象
reader = BarCodeReader()
# 读取图像
img = open('qrcode.png', 'rb').read()
# 识别二维码
data = reader.decode(img)
# 如果检测到二维码,打印内容
if data:
print("Content:", data)
```
需要注意的是,不同的库在识别二维码时可能具有不同的准确率和速度,您可以根据自己的需求选择适合的库。
相关推荐
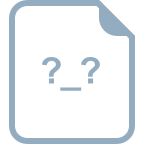
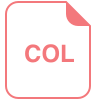
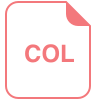
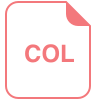
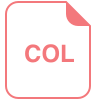
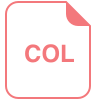









