mybatis insert返回指定字段
时间: 2023-11-26 20:58:12 浏览: 284
在 MyBatis 中进行插入操作时,可以使用 `insert` 标签来实现。如果需要返回指定字段,可以在 `insert` 标签中添加 `useGeneratedKeys` 和 `keyProperty` 属性。
例如,假设需要插入一个用户信息,并且需要返回自增主键 `id`,可以这样编写 insert 语句:
```xml
<insert id="insertUser" parameterType="User" useGeneratedKeys="true" keyProperty="id">
INSERT INTO user (username, password, email)
VALUES (#{username}, #{password}, #{email})
</insert>
```
其中,`useGeneratedKeys` 属性设置为 `true`,表示使用数据库的自增主键生成策略;`keyProperty` 属性设置为 `id`,表示将自动生成的主键值设置到 `User` 对象的 `id` 属性中。
执行插入操作后,可以通过 `User` 对象的 `getId()` 方法获取返回的自增主键值。
相关问题
mybatis insert返回主键id
在MyBatis中,插入数据并获取主键ID通常涉及到动态SQL或者使用一些特殊的插件。当你执行一个INSERT操作并希望获取新生成的主键时,可以这样做:
1. **通过结果映射**(Result Mapping):在Mapper XML文件中,对于期望自动增长的字段,如`<insert id="insertWithGeneratedKey" parameterType="com.example.YourEntity">...</insert>`,你可以设置一个结果属性`resultType="java.lang.Integer"`,并在插入语句后添加`RETURNING id`这样的后缀,MyBatis会在后台数据库驱动层面处理这个请求。
```xml
<insert id="insertWithGeneratedKey" parameterType="YourEntity" useGeneratedKeys="true" keyProperty="id">
INSERT INTO your_table (column1, column2) VALUES (#{value1}, #{value2}) RETURNING id;
</insert>
```
2. **通过回调通知**(Callback Notification):如果你使用的是MyBatis Plus等框架,它们可能提供了一种执行完插入操作后自动获取主键的方法,例如`insertOrUpdate()`,它会在完成后调用自定义的回调方法传递主键值。
3. **显式查询获取**:执行完插入操作后,你可以再发送一条SELECT语句来获取刚刚插入的新记录的主键,但这通常不是最佳实践,因为它会增加额外的数据库交互。
无论哪种方式,记住要在持久层代码中处理可能的null值,并确保你的数据库配置支持自动增长的主键策略。
mybatis insert中判断如果字段属性值为null则赋值null
可以在 MyBatis 的 INSERT 语句中使用 `<if>` 标签来判断一个属性值是否为 null,如果是,则赋值为 null。具体实现如下:
```xml
<insert id="insertUser" parameterType="User">
INSERT INTO user(name, age, address)
VALUES(
#{name},
<if test="age == null">null</if>
<if test="age != null">#{age}</if>
,
#{address}
)
</insert>
```
这里使用了 `<if>` 标签来判断 age 是否为 null。如果 age 为 null,那么就插入 null 值;否则,插入 age 的值。注意,在使用 `<if>` 标签时,需要将整个值插入到 `<if>` 标签内部,而不是只插入 null 值。
阅读全文
相关推荐
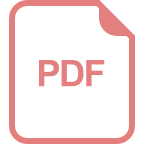
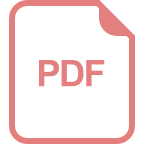
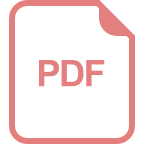
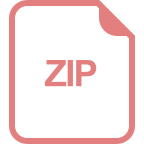











