在Arcgis中使用代码绘制动态路线图
时间: 2024-05-03 13:20:52 浏览: 8
以下是使用Python代码在ArcGIS中绘制动态路线图的步骤:
1. 导入必要的模块和库:
```python
import arcpy
from arcpy import env
from arcpy import da
import time
```
2. 设置环境变量和工作空间:
```python
env.overwriteOutput = True
env.workspace = "C:/data"
```
3. 定义输入和输出路径:
```python
in_table = "C:/data/routes.csv"
out_feature_class = "C:/data/routes.shp"
out_layer = "routes_layer"
```
4. 创建空的线要素类:
```python
arcpy.CreateFeatureclass_management(env.workspace, out_feature_class, "POLYLINE")
```
5. 添加字段:
```python
arcpy.AddField_management(out_feature_class, "ROUTE_ID", "TEXT", field_length=50)
arcpy.AddField_management(out_feature_class, "TRAVEL_TIME", "DOUBLE")
```
6. 使用InsertCursor创建要素:
```python
cursor = arcpy.da.InsertCursor(out_feature_class, ["SHAPE@", "ROUTE_ID", "TRAVEL_TIME"])
with open(in_table, "r") as f:
for line in f:
# 解析CSV文件中的数据
route_id, travel_time, coords = line.strip().split(",")
coords = [map(float, coord.split()) for coord in coords.split(";")]
# 创建Polyline对象
polyline = arcpy.Polyline(arcpy.Array([arcpy.Point(*coord) for coord in coords]))
# 插入要素
cursor.insertRow([polyline, route_id, float(travel_time)])
# 暂停1秒,以便动态显示
time.sleep(1)
del cursor
```
7. 创建图层并添加要素:
```python
arcpy.MakeFeatureLayer_management(out_feature_class, out_layer)
arcpy.mapping.AddLayer(df, arcpy.mapping.Layer(out_layer), "TOP")
```
8. 设置符号和标注:
```python
layer = arcpy.mapping.ListLayers(mxd, out_layer, df)[0]
sym = layer.symbology
sym.renderer.symbol.applySymbolFromGallery("Transportation", "Railroad 2")
layer.symbology = sym
layer.showLabels = True
```
9. 美化地图:
```python
df.extent = layer.getSelectedExtent()
df.scale = 50000
arcpy.RefreshActiveView()
arcpy.RefreshTOC()
```
10. 完整代码:
```python
import arcpy
from arcpy import env
from arcpy import da
import time
env.overwriteOutput = True
env.workspace = "C:/data"
in_table = "C:/data/routes.csv"
out_feature_class = "C:/data/routes.shp"
out_layer = "routes_layer"
arcpy.CreateFeatureclass_management(env.workspace, out_feature_class, "POLYLINE")
arcpy.AddField_management(out_feature_class, "ROUTE_ID", "TEXT", field_length=50)
arcpy.AddField_management(out_feature_class, "TRAVEL_TIME", "DOUBLE")
cursor = arcpy.da.InsertCursor(out_feature_class, ["SHAPE@", "ROUTE_ID", "TRAVEL_TIME"])
with open(in_table, "r") as f:
for line in f:
route_id, travel_time, coords = line.strip().split(",")
coords = [map(float, coord.split()) for coord in coords.split(";")]
polyline = arcpy.Polyline(arcpy.Array([arcpy.Point(*coord) for coord in coords]))
cursor.insertRow([polyline, route_id, float(travel_time)])
time.sleep(1)
del cursor
mxd = arcpy.mapping.MapDocument("CURRENT")
df = arcpy.mapping.ListDataFrames(mxd)[0]
arcpy.MakeFeatureLayer_management(out_feature_class, out_layer)
arcpy.mapping.AddLayer(df, arcpy.mapping.Layer(out_layer), "TOP")
layer = arcpy.mapping.ListLayers(mxd, out_layer, df)[0]
sym = layer.symbology
sym.renderer.symbol.applySymbolFromGallery("Transportation", "Railroad 2")
layer.symbology = sym
layer.showLabels = True
df.extent = layer.getSelectedExtent()
df.scale = 50000
arcpy.RefreshActiveView()
arcpy.RefreshTOC()
```
相关推荐
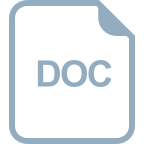
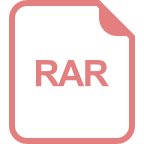










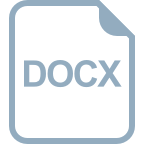
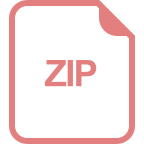
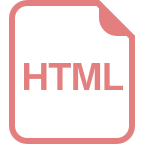