vue.js梁灏购物车
时间: 2024-12-26 21:27:21 浏览: 0
### 使用 Vue.js 实现购物车功能
#### 创建 HTML 结构
为了构建基于 Vue.js 的购物车应用,首先需要设置好项目的HTML结构。这包括引入必要的Vue脚本以及定义用于展示商品列表和购物车的主要容器。
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>购物车</title>
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<div id="app" v-cloak>
<!-- 商品列表 -->
<ul>
<li v-for="(item, index) in items" :key="index">
{{ item.name }} - ¥{{ item.price }}
<button @click="addToCart(index)">加入购物车</button>
</li>
</ul>
<!-- 购物车详情 -->
<h3>我的购物车</h3>
<ul>
<li v-for="(cartItem, idx) in cartItems" :key="idx">
{{ cartItem.name }} × {{ cartItem.quantity }} = ¥{{ (cartItem.price * cartItem.quantity).toFixed(2) }}
<button @click="removeFromCart(idx)">移除</button>
</li>
</ul>
总价: ¥{{ totalPrice.toFixed(2) }}
</div>
<script src="https://cdn.bootcss.com/vue/2.2.2/vue.min.js"></script>
<script src="index.js"></script>
</body>
</html>
```
此部分展示了如何搭建一个简单的网页框架并加载所需的外部资源[^3]。
#### 编写 JavaScript 逻辑
接下来,在`<script>`标签内部编写JavaScript代码来初始化Vue实例,并处理添加到购物车的操作:
```javascript
new Vue({
el: '#app',
data() {
return {
items: [
{ name: '苹果', price: 5 },
{ name: '香蕉', price: 3 }
],
cartItems: []
};
},
computed: {
totalPrice() {
let total = 0;
this.cartItems.forEach(item => {
total += item.price * item.quantity;
});
return total;
}
},
methods: {
addToCart(index) {
const selectedItem = Object.assign({}, this.items[index], { quantity: 1 });
// 查找是否已存在相同项
const existingIndex = this.cartItems.findIndex(cartItem =>
cartItem.name === selectedItem.name);
if (existingIndex >= 0) {
// 如果已经存在于购物车内,则增加数量
this.cartItems[existingIndex].quantity++;
} else {
// 否则新增一项至购物车数组中
this.cartItems.push(selectedItem);
}
},
removeFromCart(index) {
if (this.cartItems[index].quantity > 1) {
this.cartItems[index].quantity--;
} else {
this.cartItems.splice(index, 1);
}
}
}
});
```
上述代码片段实现了两个主要的功能——向购物车添加商品和从购物车删除商品。同时计算了当前购物车内的总价。
阅读全文
相关推荐
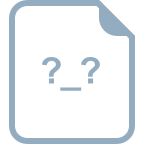
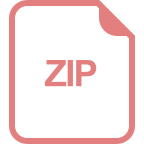
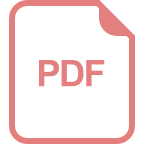
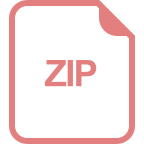
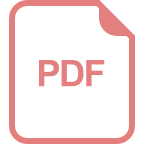
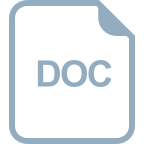
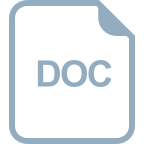
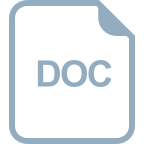
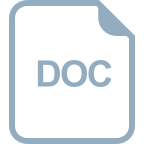
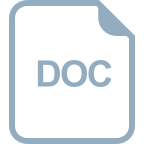

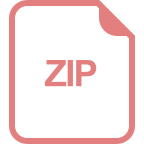
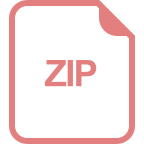
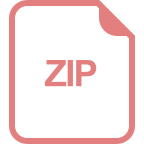
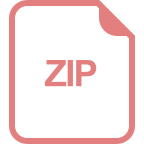
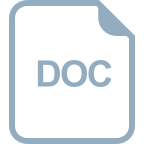
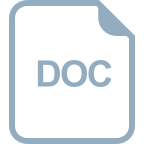
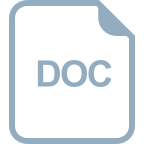