react antd form 校验替换特殊字符,特殊字符不可以输入
时间: 2024-10-23 21:03:21 浏览: 35
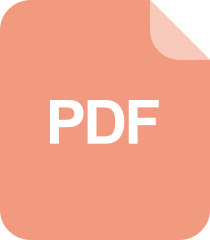
在react-antd中弹出层form内容传递给父组件的操作
在React中使用Ant Design Form进行表单校验,若希望阻止用户输入特殊字符并替换为默认值,你可以结合`Input`组件的`onKeyDown`事件和自定义验证规则来实现。首先,创建一个`replaceSpecialChar`函数,用于过滤掉特殊字符:
```jsx
const replaceSpecialChar = (event, allowedChars = '') => {
const key = event.key;
const keyValue = String.fromCharCode(key);
if (!allowedChars.includes(keyValue)) {
event.preventDefault(); // 阻止键盘输入
event.target.value = keyValue === ' ' ? '' : allowedChars; // 替换为允许的字符或空格
}
};
```
然后,在`Form.Item`内配置`Input`组件和校验规则:
```jsx
import { Form, Input, Button } from 'antd';
const CustomForm = () => {
const [allowedChars, setAllowedChars] = useState(''); // 可以根据需求动态设置允许的字符
const handleAllowedCharsChange = (e) => {
setAllowedChars(e.target.value);
};
const onInputChange = (event) => {
replaceSpecialChar(event, allowedChars);
};
return (
<Form>
{/* 添加允许字符的选择框 */}
<Form.Item
label="Allowed Characters"
name="allowedChars"
value={allowedChars}
onChange={handleAllowedCharsChange}
>
<Input placeholder="Enter allowed characters" />
</Form.Item>
<FormItem
name="inputField"
rules={[
{ validator: (values, callback) => {
const { inputField } = values;
if (!allowedChars || !inputField) {
callback(); // 如果未设定允许字符,直接通过校验
} else if (inputField.includes(restrictedCharacters(allowedChars))) {
callback(new Error('Please enter only allowed characters.'));
} else {
callback();
}
}, trigger: 'change' }
]}
extra={<small>Only allow the characters you specified</small>}
>
<Input
onKeyDown={onInputChange}
placeholder="Enter your text without special characters"
/>
</FormItem>
{/* ...其他表单元素... */}
</Form>
);
};
export default CustomForm;
```
这里,`onInputChange`会在用户输入时实时检查并替换特殊字符。`rules`部分则在`change`事件触发时校验输入内容。
阅读全文
相关推荐





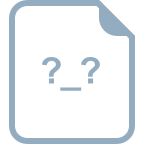
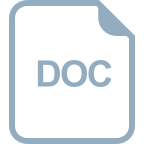
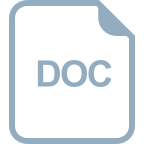
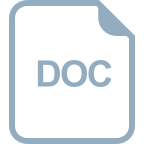

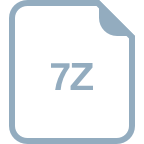
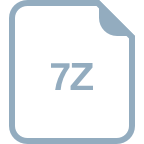