如何用python代码实现支持向量机模型,具体的步骤如下1,对数据进行基本统计分析,对数据正确划分训练集、测试集。2,使用验证集对分类器调参,获取最优模型( 对获得的最优模型,计算测试集上的Acc值、Precision值、Recall值、PR曲线、F1值、TPR值、FPR值、ROC曲线、AUC值。尝试对数据进行预处理
时间: 2023-12-28 07:11:31 浏览: 182
好的,下面是使用Python实现支持向量机模型的具体步骤:
1. 导入必要的库和数据集:
```python
import pandas as pd
import numpy as np
from sklearn.model_selection import train_test_split
from sklearn.svm import SVC
from sklearn.metrics import accuracy_score, precision_score, recall_score, f1_score, roc_auc_score, roc_curve, auc, confusion_matrix
import matplotlib.pyplot as plt
# 读取数据集
data = pd.read_csv('data.csv')
# 获取特征和标签
X = data.iloc[:,:-1]
y = data.iloc[:,-1]
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
```
2. 进行数据预处理,如标准化、归一化等:
```python
# 数据标准化
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
X_train = scaler.fit_transform(X_train)
X_test = scaler.transform(X_test)
```
3. 训练支持向量机模型并进行分类器调参:
```python
# 训练支持向量机模型
svc = SVC(kernel='linear', C=1)
svc.fit(X_train, y_train)
# 使用验证集进行模型调优
from sklearn.model_selection import GridSearchCV
param_grid = {'C': [0.1, 1, 10], 'kernel': ['linear', 'rbf', 'poly']}
grid_search = GridSearchCV(SVC(), param_grid, cv=5)
grid_search.fit(X_train, y_train)
# 获取最优模型
best_svc = grid_search.best_estimator_
```
4. 在测试集上计算模型的各项指标:
```python
# 在测试集上进行预测
y_pred = best_svc.predict(X_test)
# 计算准确率、精确率、召回率、F1值、AUC值
acc = accuracy_score(y_test, y_pred)
precision = precision_score(y_test, y_pred)
recall = recall_score(y_test, y_pred)
f1 = f1_score(y_test, y_pred)
auc = roc_auc_score(y_test, y_pred)
# 绘制ROC曲线
fpr, tpr, thresholds = roc_curve(y_test, y_pred)
roc_auc = auc(fpr, tpr)
plt.plot(fpr, tpr, label='ROC curve (area = %0.2f)' % roc_auc)
plt.plot([0, 1], [0, 1], 'k--')
plt.xlim([0.0, 1.0])
plt.ylim([0.0, 1.05])
plt.xlabel('False Positive Rate')
plt.ylabel('True Positive Rate')
plt.title('Receiver operating characteristic')
plt.legend(loc="lower right")
plt.show()
```
5. 输出模型的各项指标以及混淆矩阵:
```python
# 输出模型的各项指标
print('Accuracy:', acc)
print('Precision:', precision)
print('Recall:', recall)
print('F1 Score:', f1)
print('AUC Score:', auc)
# 输出混淆矩阵
cm = confusion_matrix(y_test, y_pred)
print('Confusion Matrix:\n', cm)
```
以上就是使用Python实现支持向量机模型的具体步骤,希望对你有所帮助。
阅读全文
相关推荐
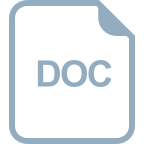
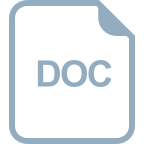
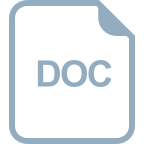

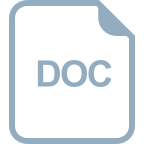
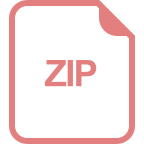
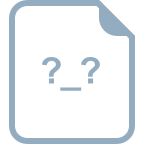
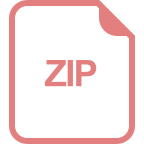
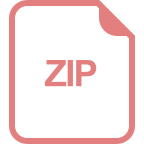
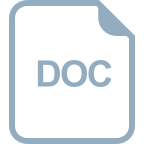
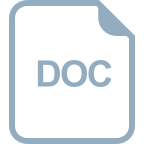
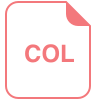
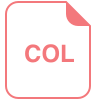
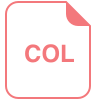
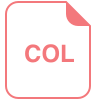
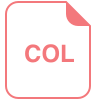


