用c++编写程序实现走迷宫小游戏
时间: 2023-08-13 13:31:09 浏览: 67
好的,下面是一个用C++编写的走迷宫小游戏:
```c++
#include <iostream>
#include <vector>
#include <string>
using namespace std;
// 迷宫地图
const vector<string> maze{
"##########",
"#S #",
"# #### #",
"# # #",
"# # ####",
"# # #",
"# #### #",
"# #",
"# ######",
"# #E #",
"##########"
};
int main()
{
// 迷宫地图的行数和列数
const int rows = maze.size();
const int cols = maze[0].size();
// 玩家的起始位置
int x = 1;
int y = 1;
// 玩家的体力值
int health = 10;
// 游戏主循环
while (true)
{
// 打印当前迷宫地图和玩家状态
cout << endl;
for (int i = 0; i < rows; i++)
{
for (int j = 0; j < cols; j++)
{
if (i == x && j == y)
{
cout << "O";
}
else
{
cout << maze[i][j];
}
}
cout << endl;
}
cout << "Health: " << health << endl;
// 检查是否到达终点
if (maze[x][y] == 'E')
{
cout << "You win!" << endl;
break;
}
// 检查是否体力值耗尽
if (health <= 0)
{
cout << "You lose!" << endl;
break;
}
// 获取玩家输入
char input;
cout << "Enter w, s, a, or d to move: ";
cin >> input;
// 移动玩家
int new_x = x, new_y = y;
if (input == 'w')
{
new_x--;
}
else if (input == 's')
{
new_x++;
}
else if (input == 'a')
{
new_y--;
}
else if (input == 'd')
{
new_y++;
}
// 检查移动是否合法
if (new_x < 0 || new_x >= rows || new_y < 0 || new_y >= cols)
{
cout << "Invalid move!" << endl;
}
else if (maze[new_x][new_y] == '#')
{
cout << "You hit a wall!" << endl;
}
else
{
// 更新玩家位置和体力值
x = new_x;
y = new_y;
health--;
if (maze[x][y] == 'H')
{
cout << "You found a health pack, +3 health!" << endl;
health += 3;
}
}
}
return 0;
}
```
这个程序用了一个简单的二维数组来表示迷宫地图,用变量`x`和`y`表示玩家的位置,用变量`health`表示玩家的体力值。在游戏主循环中,程序打印出当前的迷宫地图和玩家状态,然后等待玩家输入,并根据输入移动玩家。在移动玩家时,程序检查移动是否合法,如果合法则更新玩家位置和体力值。当玩家到达终点或体力值耗尽时,游戏结束。
相关推荐
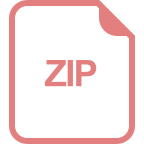
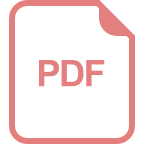














