用java写一段http文件上传
时间: 2023-06-03 19:07:17 浏览: 124
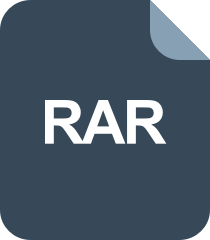
Java web文件上传
代码怎么写?
好的,以下是一个简单的Java文件上传的示例代码:
```
import java.io.File;
import java.io.IOException;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.UUID;
public class FileUploader {
public static void upload(String urlString, String filePath) throws IOException {
// 生成随机边界
String boundary = UUID.randomUUID().toString();
// 创建URL对象
URL url = new URL(urlString);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
// 设置请求方法为POST
connection.setRequestMethod("POST");
// 设置请求属性
connection.setRequestProperty("Content-Type", "multipart/form-data; boundary=" + boundary);
// 打开连接,开始上传文件
connection.setDoOutput(true);
connection.connect();
// 获取文件名
File file = new File(filePath);
String fileName = file.getName();
// 构建请求体
StringBuilder builder = new StringBuilder();
builder.append("--").append(boundary).append("\r\n");
builder.append("Content-Disposition: form-data; name=\"file\"; filename=\"").append(fileName).append("\"\r\n");
builder.append("Content-Type: application/octet-stream\r\n\r\n");
byte[] headerBytes = builder.toString().getBytes();
// 把请求头写入输出流
connection.getOutputStream().write(headerBytes);
// 读取文件,把文件内容写入输出流
FileInputStream inputStream = new FileInputStream(file);
byte[] buffer = new byte[4096];
int bytesRead = -1;
while ((bytesRead = inputStream.read(buffer)) != -1) {
connection.getOutputStream().write(buffer, 0, bytesRead);
}
inputStream.close();
// 结束请求体
builder = new StringBuilder();
builder.append("\r\n--").append(boundary).append("--\r\n");
byte[] footerBytes = builder.toString().getBytes();
connection.getOutputStream().write(footerBytes);
// 获取响应
int responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
System.out.println("上传成功!");
} else {
System.out.println("上传失败!");
}
connection.disconnect();
}
public static void main(String[] args) throws IOException {
String urlString = "http://www.example.com/upload";
String filePath = "C:/example.txt";
upload(urlString, filePath);
}
}
```
这个示例代码可以将本地的一个文件上传到指定的URL地址。如果文件上传成功,就会在控制台输出"上传成功!",否则输出"上传失败!"。注意,这个代码中的boundary是一个随机生成的字符串,用来分割不同的请求参数。在写请求体时,要按照multipart/form-data格式的规定来构造RequestBody。如果上传的文件很大,可以考虑使用分段上传,以减少内存占用。
阅读全文
相关推荐


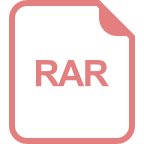


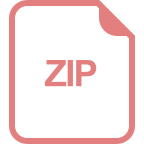
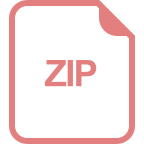
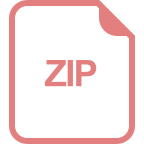
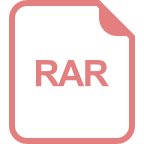
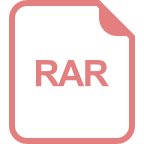
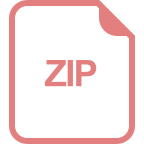




