用dosbox实现1到100求和,并以字符串形式显示,再实现直接显屏
时间: 2023-12-14 18:38:07 浏览: 225
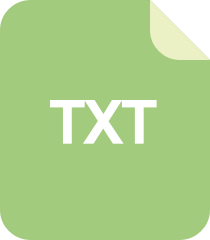
汇编实现1到100求和
首先,我们需要编写一个 DOS 下的程序来实现 1 到 100 求和,并将结果以字符串形式显示出来。
以下是一个简单的汇编程序,可以实现这个功能:
```
.model tiny
.code
org 100h
start:
mov ax, 0 ; 初始化累加器
mov cx, 100 ; 循环次数
mov bx, offset result ; 存放结果的地址
sum_loop:
add ax, cx ; 累加
loop sum_loop ; 循环
call num2str ; 将结果转换为字符串
mov ah, 09h ; 调用 DOS 中断显示字符串
mov dx, offset result_str
int 21h
mov ah, 4ch ; 返回 DOS
int 21h
num2str:
mov si, offset result ; 存放结果的地址
mov di, offset result_str ; 存放结果字符串的地址
mov bx, 10 ; 除数
num2str_loop:
xor dx, dx ; 清除余数
div bx ; 除法运算
add dl, '0' ; 将余数转换为 ASCII 码
mov [di], dl ; 存储到结果字符串中
inc di ; 移动指针
cmp ax, 0 ; 判断是否已经除尽
jne num2str_loop
mov byte ptr [di], '$' ; 字符串结尾
ret
result dw ?
result_str db 10 dup ('$')
end start
```
这个程序使用了两个变量:`result` 存放结果,`result_str` 存放结果字符串。在主过程中,使用循环将 1 到 100 累加起来,并将结果存放到 `result` 变量中。然后调用 `num2str` 过程将结果转换为字符串,并存储到 `result_str` 中。最后使用 DOS 中断显示字符串。
接下来,我们需要使用 DOSBox 来运行这个程序。首先,需要将程序保存到一个文件中,例如 `sum.asm`。然后,在 DOSBox 中执行以下命令编译和运行程序:
```
tasm sum.asm
tlink sum.obj
sum.exe
```
这样,程序就会在 DOSBox 中运行,并将结果以字符串形式显示出来。
如果需要直接显示在屏幕上,可以修改程序,调用 DOS 中断显示字符(`int 10h`)来逐个输出结果字符串中的字符。以下是修改后的程序:
```
.model tiny
.code
org 100h
start:
mov ax, 0 ; 初始化累加器
mov cx, 100 ; 循环次数
sum_loop:
add ax, cx ; 累加
loop sum_loop ; 循环
call num2str ; 将结果转换为字符串
mov si, offset result_str ; 存放结果字符串的地址
print_loop:
mov ah, 0eh ; 调用 DOS 中断显示字符
mov al, [si]
cmp al, '$'
je print_end
int 10h
inc si
jmp print_loop
print_end:
mov ah, 4ch ; 返回 DOS
int 21h
num2str:
mov si, offset result ; 存放结果的地址
mov di, offset result_str ; 存放结果字符串的地址
mov bx, 10 ; 除数
num2str_loop:
xor dx, dx ; 清除余数
div bx ; 除法运算
add dl, '0' ; 将余数转换为 ASCII 码
mov [di], dl ; 存储到结果字符串中
inc di ; 移动指针
cmp ax, 0 ; 判断是否已经除尽
jne num2str_loop
mov byte ptr [di], '$' ; 字符串结尾
ret
result dw ?
result_str db 10 dup ('$')
end start
```
这个程序将结果字符串逐个输出到屏幕上,直到遇到字符串结尾符 `$`。最后调用 DOS 中断返回操作系统。
在 DOSBox 中执行命令编译和运行程序:
```
tasm sum.asm
tlink sum.obj
sum.exe
```
这样,程序就会在 DOSBox 中运行,并直接显示结果。
阅读全文
相关推荐
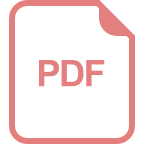
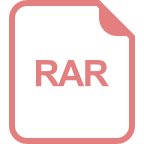
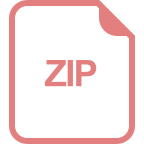
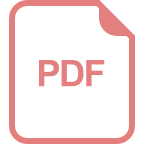
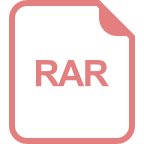
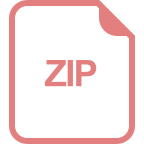
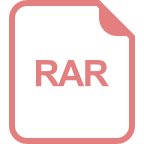
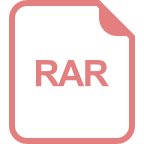
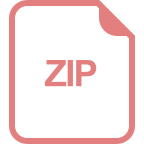
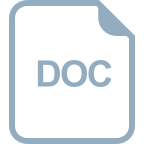
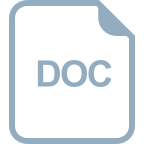
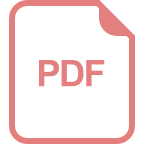
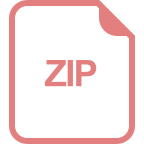
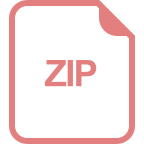