如何修改训练代码使得可以读训练的GFLOPS和latency
时间: 2023-12-29 10:04:29 浏览: 28
要读取训练的GFLOPS和latency,需要在训练代码中添加相应的代码。以下是一些可能的方法:
1. 使用PyTorch的Profiler模块来获取GFLOPS和latency。在训练代码中,可以使用Profiler模块来记录每个操作的运行时间和计算量,并计算出GFLOPS和latency。例如:
```python
import torch.profiler as profiler
with profiler.profile(record_shapes=True) as prof:
# 训练过程
for epoch in range(num_epochs):
for data in train_loader:
# 前向传播、反向传播、更新参数等操作
# ...
prof.export_chrome_trace("trace.json")
print(prof.key_averages().table(sort_by="cpu_time_total", row_limit=10))
```
这段代码会使用Profiler模块记录训练过程中的每个操作,并将结果导出到Chrome Trace格式的文件中。然后可以使用Profiler模块提供的函数来获取GFLOPS和latency等信息。
2. 使用第三方库来获取GFLOPS和latency。还可以使用一些第三方库来获取GFLOPS和latency等信息,例如Flops-counter.pytorch和PyTorch-Benchmark。这些库可以通过分析模型的结构来估算模型的计算量,并计算出GFLOPS和latency等信息。
```python
from thop import profile
# 定义模型
model = ...
# 计算模型的计算量和参数量
input = torch.randn(batch_size, 3, 224, 224)
flops, params = profile(model, inputs=(input,))
print(flops / 1e9, params / 1e6)
# 使用PyTorch Benchmark来计算latency
from torch.utils.benchmark import Timer
# 定义输入
input = torch.randn(batch_size, 3, 224, 224)
# 定义模型和输入
model = ...
model.eval()
model = model.cuda()
input = input.cuda()
# 运行模型,计算latency
timer = Timer()
timer.start()
with torch.no_grad():
for i in range(num_iterations):
output = model(input)
timer.stop()
print(timer.elapsed_secs / num_iterations)
```
这段代码会使用Flops-counter.pytorch库来计算模型的计算量和参数量,并使用PyTorch Benchmark来计算模型的latency。请注意,这些库可能需要对模型进行修改才能正确计算GFLOPS和latency。
相关推荐
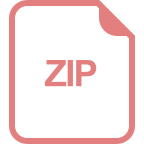
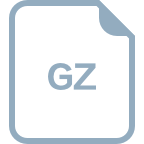
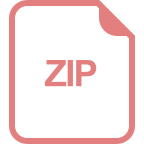














