用c语言编写一个正规表达式编辑器,用LL(1)分析实现并给出测试用例
时间: 2024-03-26 07:40:03 浏览: 67
正则表达式是一种强大的文本模式匹配语言,可以用于匹配、搜索和替换字符串。下面是一个用C语言实现正则表达式编辑器的简单示例,使用LL(1)分析器实现。
首先,需要定义一个规则集合,包含所有的正则表达式语法规则。例如:
```
expr -> term '|' expr
| term
term -> factor term
| factor
factor -> atom '*' factor
| atom '+' factor
| atom '?'
| atom
atom -> '(' expr ')'
| char
char -> 'a' | 'b' | ...
```
接着,需要编写一个LL(1)分析器,根据上述规则集合解析输入的正则表达式,并生成相应的语法树。这可以通过递归下降分析器来实现。例如:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
/* Token types */
enum {
TOK_EOF,
TOK_PIPE,
TOK_STAR,
TOK_PLUS,
TOK_QUESTION,
TOK_LPAREN,
TOK_RPAREN,
TOK_CHAR
};
/* Token structure */
typedef struct {
int type;
char value;
} Token;
/* Input string */
char *input;
/* Current position in input */
int pos;
/* Current token */
Token token;
/* Forward declaration */
void expr();
void term();
void factor();
void atom();
/* Get the next character from input */
char next() {
return input[pos++];
}
/* Peek the next character from input */
char peek() {
return input[pos];
}
/* Create a new token */
Token new_token(int type, char value) {
Token token;
token.type = type;
token.value = value;
return token;
}
/* Get the next token from input */
Token next_token() {
char c = next();
switch (c) {
case '|': return new_token(TOK_PIPE, '|');
case '*': return new_token(TOK_STAR, '*');
case '+': return new_token(TOK_PLUS, '+');
case '?': return new_token(TOK_QUESTION, '?');
case '(': return new_token(TOK_LPAREN, '(');
case ')': return new_token(TOK_RPAREN, ')');
default:
if (isalpha(c)) {
return new_token(TOK_CHAR, c);
} else {
printf("Invalid character %c\n", c);
exit(1);
}
}
}
/* Match a token */
void match(int type) {
if (token.type == type) {
token = next_token();
} else {
printf("Error: expected %d, got %d\n", type, token.type);
exit(1);
}
}
/* Parse an expression */
void expr() {
term();
if (token.type == TOK_PIPE) {
match(TOK_PIPE);
expr();
}
}
/* Parse a term */
void term() {
factor();
if (token.type != TOK_PIPE && token.type != TOK_EOF && token.type != TOK_RPAREN) {
term();
}
}
/* Parse a factor */
void factor() {
atom();
if (token.type == TOK_STAR) {
match(TOK_STAR);
factor();
} else if (token.type == TOK_PLUS) {
match(TOK_PLUS);
factor();
} else if (token.type == TOK_QUESTION) {
match(TOK_QUESTION);
}
}
/* Parse an atom */
void atom() {
if (token.type == TOK_LPAREN) {
match(TOK_LPAREN);
expr();
match(TOK_RPAREN);
} else if (token.type == TOK_CHAR) {
match(TOK_CHAR);
} else {
printf("Error: expected atom, got %d\n", token.type);
exit(1);
}
}
/* Test the LL(1) parser */
void test() {
input = "a(b|c)*d";
pos = 0;
token = next_token();
expr();
if (token.type != TOK_EOF) {
printf("Error: unexpected token %d\n", token.type);
exit(1);
}
printf("Valid regex\n");
}
int main() {
test();
return 0;
}
```
在上述代码中,我们首先定义了Token类型和一些常量,然后实现了一个LL(1)分析器。该分析器使用递归下降法对输入的正则表达式进行解析,并生成相应的语法树。最后,我们编写了一个test()函数来测试分析器,使用了正则表达式"a(b|c)*d"作为输入。
阅读全文
相关推荐
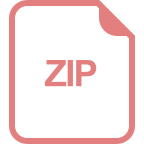
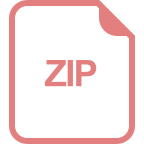
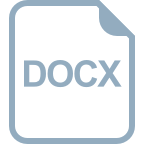
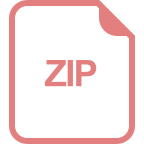
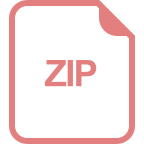
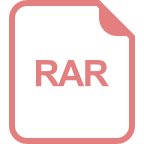
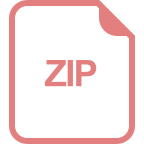
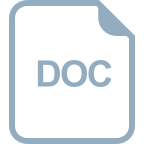
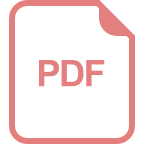
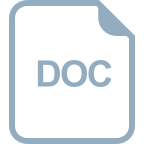
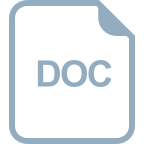
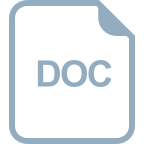


