crowdnet pytorch
时间: 2023-11-20 19:50:38 浏览: 37
CrowdNet is a deep learning model developed for crowd counting and density estimation tasks. It is typically implemented using the PyTorch framework. The model utilizes convolutional neural networks (CNNs) to learn and predict crowd densities in images or videos.
To use CrowdNet in PyTorch, you would need to download or clone the CrowdNet PyTorch implementation from a repository or source. Once you have the code, you can import the necessary modules, define the CrowdNet architecture, and train or evaluate the model using your dataset.
Here is a basic example of how you can use CrowdNet in PyTorch:
```python
import torch
import torch.nn as nn
import torch.optim as optim
from torchvision.models import vgg16
# Define the CrowdNet architecture
class CrowdNet(nn.Module):
def __init__(self):
super(CrowdNet, self).__init__()
self.vgg = vgg16(pretrained=True)
self.fc = nn.Linear(1000, 1) # Output layer for density estimation
def forward(self, x):
x = self.vgg(x)
x = self.fc(x)
return x
# Instantiate the CrowdNet model
model = CrowdNet()
# Define loss function and optimizer
criterion = nn.MSELoss()
optimizer = optim.SGD(model.parameters(), lr=0.001, momentum=0.9)
# Training loop
for epoch in range(num_epochs):
# Forward pass
outputs = model(inputs)
loss = criterion(outputs, labels)
# Backward and optimize
optimizer.zero_grad()
loss.backward()
optimizer.step()
# Print progress
if (epoch+1) % 10 == 0:
print(f"Epoch {epoch+1}/{num_epochs}, Loss: {loss.item()}")
# Evaluation
with torch.no_grad():
outputs = model(test_inputs)
# Perform evaluation metrics calculation
...
```
Please note that this is just a basic example, and depending on your specific requirements and dataset, you may need to modify the architecture, loss function, optimizer, and training loop accordingly. Also, make sure to preprocess your dataset appropriately before feeding it into the model.
I hope this helps! Let me know if you have any more questions.
相关推荐
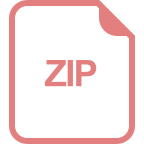














