JAVA客房管理系统连接数据库
时间: 2023-07-20 09:38:38 浏览: 138
要连接数据库,需要使用Java的JDBC API。以下是一个简单的Java程序,用于连接MySQL数据库并执行查询:
```java
import java.sql.*;
public class HotelManagementSystem {
public static void main(String[] args) {
Connection con = null;
Statement stmt = null;
ResultSet rs = null;
try {
// 加载MySQL驱动程序
Class.forName("com.mysql.jdbc.Driver");
// 连接MySQL数据库
String url = "jdbc:mysql://localhost:3306/hotel";
String user = "root";
String password = "password";
con = DriverManager.getConnection(url, user, password);
// 执行查询
stmt = con.createStatement();
rs = stmt.executeQuery("SELECT * FROM rooms");
// 处理结果集
while (rs.next()) {
int roomNumber = rs.getInt("room_number");
String roomType = rs.getString("room_type");
double price = rs.getDouble("price");
System.out.println("Room " + roomNumber + " is a " + roomType + " and costs $" + price + " per night.");
}
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
} finally {
// 关闭数据库连接
try {
if (rs != null) {
rs.close();
}
if (stmt != null) {
stmt.close();
}
if (con != null) {
con.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
在此代码中,我们首先加载MySQL驱动程序,然后使用`DriverManager.getConnection()`方法连接到MySQL数据库。接下来,我们使用`createStatement()`方法创建一个Statement对象,并使用`executeQuery()`方法执行查询。最后,我们循环遍历结果集,并将结果打印到控制台。注意,在完成查询后,我们必须关闭所有数据库对象,以释放资源。
当运行此程序时,应该会看到类似以下内容的输出:
```
Room 101 is a standard and costs $100.0 per night.
Room 102 is a deluxe and costs $150.0 per night.
Room 103 is a suite and costs $200.0 per night.
```
这是从数据库中检索出的客房信息。
阅读全文
相关推荐
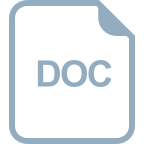
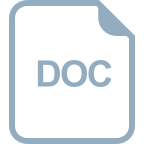
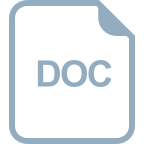

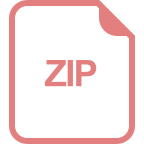
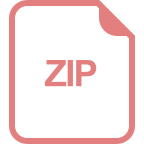
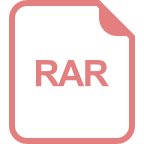
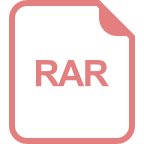
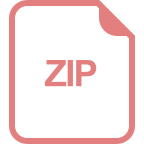
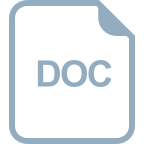
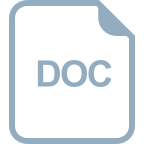


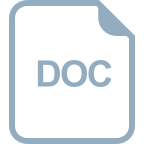
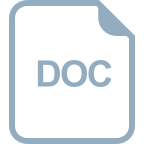
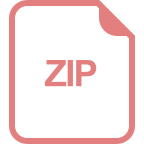
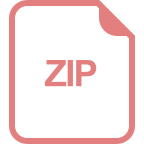
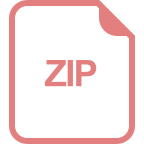
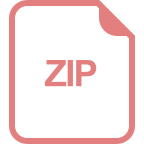