智能驾驶员模型(IDM)实现横坐标为500辆车,纵坐标为速度变化动图的python代码
时间: 2024-09-22 20:10:23 浏览: 84
智能驾驶员模型(Intelligent Driver Model, IDM)通常用于模拟道路上的车流行为,它基于车辆的行驶速度、加速度等参数来预测车辆的行为。以下是一个简单的Python代码示例,展示了如何使用IDM生成一个500辆车的动图。我们将使用`matplotlib`库来进行绘图:
```python
import numpy as np
import matplotlib.pyplot as plt
from scipy.optimize import fsolve
# IDM 参数设置
a = 1.2 # 加速度偏好
b = 2.0 # 最大安全速度减速度
T = 1.6 # 规定反应时间
S0 = 2.0 # 常规跟驰距离
d0 = 4.0 # 规定最小间隔
v0 = 80 # 初始速度(km/h)
v_max = 120 # 最大速度(km/h)
# 将参数从km/h转为m/s
a_mps = a * 3.6
b_mps = b * 3.6
T_mps = T * 3.6
S0_mps = S0 * 3.6
d0_mps = d0 * 3.6
v0_mps = v0 * 3.6
v_max_mps = v_max * 3.6
# 定义 IDM 函数
def idm_func(v, t, s, v0, v_max, T, a, b, d0):
delta_v = (v_max - v) / v_max * a * np.exp(-b * (t-T))
v_next = max(v + delta_v, v_min)
s_next = s + v * (t - T) + d0
return v_next - v, s_next - s
# 创建500辆车的位置和速度数组
num_vehicles = 500
positions = np.zeros(num_vehicles)
velocities = np.full(num_vehicles, v0_mps)
# 设置时间范围和时间步长
total_time = 10.0 # 秒
time_step = 0.01 # 秒
times = np.arange(0, total_time + time_step, time_step)
# 解决每个时间步骤的IDM方程
for t in times[1:]:
# 对于每一辆车,更新速度和位置
for i in range(num_vehicles):
s = positions[i]
sol = fsolve(idm_func, velocities[i], args=(t, s, v0_mps, v_max_mps, T_mps, a_mps, b_mps, d0_mps))
velocities[i] = sol[0]
positions[i] = s + velocities[i] * time_step
# 绘制动图
plt.figure(figsize=(12, 6))
plt.plot(positions, velocities, '.')
plt.xlabel('Position (m)')
plt.ylabel('Speed (m/s)')
plt.title('IDM Simulation of 500 Vehicles')
plt.grid(True)
plt.show()
阅读全文
相关推荐
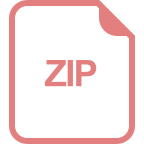
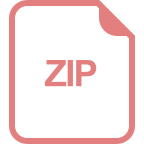
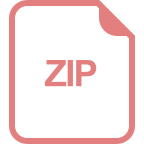
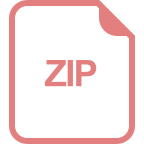
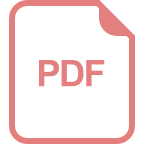
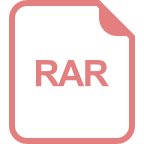
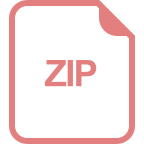
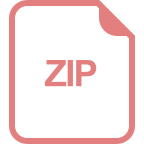
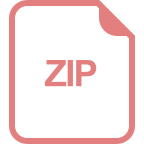
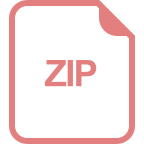
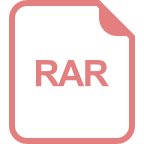
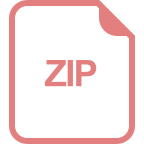
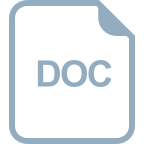
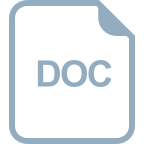