银行管理系统c++ 用虚函数和纯虚函数写
时间: 2023-12-27 16:16:18 浏览: 87
以下是一个银行账户管理系统的代码示例,使用了虚函数和纯虚函数:
```c++
#include <iostream>
#include <string>
using namespace std;
class Account {
protected:
string name;
int balance;
public:
Account(string n, int b) : name(n), balance(b) {}
virtual void deposit(int amount) {
balance += amount;
cout << "Deposit " << amount << " successfully." << endl;
}
virtual bool withdraw(int amount) {
if (balance >= amount) {
balance -= amount;
cout << "Withdraw " << amount << " successfully." << endl;
return true;
}
else {
cout << "Withdraw " << amount << " failed." << endl;
return false;
}
}
virtual void show() {
cout << "Name: " << name << endl;
cout << "Balance: " << balance << endl;
}
};
class SavingAccount : public Account {
protected:
double rate;
public:
SavingAccount(string n, int b, double r) : Account(n, b), rate(r) {}
virtual void deposit(int amount) {
balance += amount;
cout << "Deposit " << amount << " successfully." << endl;
balance += balance * rate;
cout << "Interest added." << endl;
}
};
class CheckingAccount : public Account {
protected:
int fee;
public:
CheckingAccount(string n, int b, int f) : Account(n, b), fee(f) {}
virtual bool withdraw(int amount) {
if (balance >= amount + fee) {
balance -= amount + fee;
cout << "Withdraw " << amount << " successfully." << endl;
cout << "Fee " << fee << " charged." << endl;
return true;
}
else {
cout << "Withdraw " << amount << " failed." << endl;
return false;
}
}
};
int main() {
Account* a1 = new SavingAccount("Tom", 1000, 0.01);
Account* a2 = new CheckingAccount("Jerry", 500, 10);
a1->deposit(100);
a1->show();
a2->withdraw(200);
a2->show();
delete a1;
delete a2;
return 0;
}
```
在上面的代码中,`Account` 是一个基类,`SavingAccount` 和 `CheckingAccount` 都是它的派生类。`Account` 中定义了三个虚函数 `deposit()`、`withdraw()` 和 `show()`,并且 `withdraw()` 被声明为纯虚函数。`SavingAccount` 和 `CheckingAccount` 都重写了 `deposit()` 和 `withdraw()` 函数,而 `show()` 函数继承了 `Account` 中的实现。
在 `main()` 函数中,我们创建了一个 `SavingAccount` 对象和一个 `CheckingAccount` 对象,并通过基类指针调用它们的虚函数。由于 `withdraw()` 是纯虚函数,所以我们不能创建 `Account` 对象,只能通过派生类来创建对象。
阅读全文
相关推荐
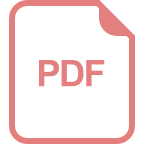
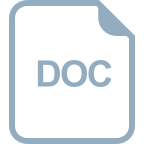
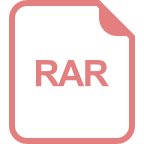
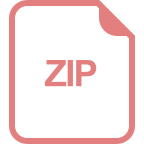

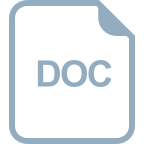
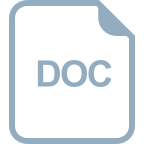
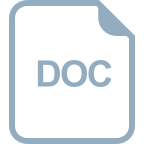
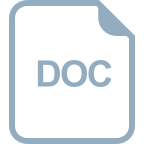
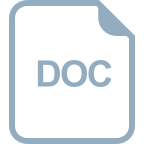
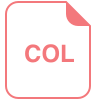
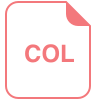
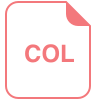
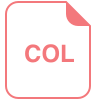
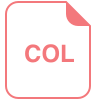
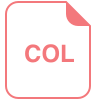
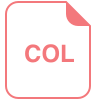