C# DataGridView绑定List对象时,利用BindingList来实现增删查改
时间: 2024-04-22 17:22:11 浏览: 189
当你要绑定List对象时,可以使用BindingList<T>来实现增删查改。
首先,你需要创建一个BindingList<T>对象并将其绑定到DataGridView控件上。在添加或删除数据时,你需要操作BindingList<T>对象,而不是List<T>对象。这样可以确保DataGridView控件能正确地显示数据。
下面是一个简单的例子,展示如何使用BindingList<T>绑定List对象并实现增删查改:
```csharp
using System.Collections.Generic;
using System.ComponentModel;
using System.Windows.Forms;
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
}
public partial class Form1 : Form
{
private BindingList<Person> people = new BindingList<Person>();
public Form1()
{
InitializeComponent();
// 将BindingList对象绑定到DataGridView控件上
dataGridView1.DataSource = people;
// 添加一些数据
people.Add(new Person { Name = "Tom", Age = 20 });
people.Add(new Person { Name = "Mary", Age = 25 });
people.Add(new Person { Name = "John", Age = 30 });
}
private void btnAdd_Click(object sender, EventArgs e)
{
// 添加一条数据
people.Add(new Person { Name = txtName.Text, Age = int.Parse(txtAge.Text) });
}
private void btnDelete_Click(object sender, EventArgs e)
{
// 删除选中的行
foreach (DataGridViewRow row in dataGridView1.SelectedRows)
{
if (!row.IsNewRow)
{
people.RemoveAt(row.Index);
}
}
}
private void btnUpdate_Click(object sender, EventArgs e)
{
// 修改选中的行
foreach (DataGridViewRow row in dataGridView1.SelectedRows)
{
if (!row.IsNewRow)
{
Person person = people[row.Index];
person.Name = txtName.Text;
person.Age = int.Parse(txtAge.Text);
}
}
}
private void dataGridView1_SelectionChanged(object sender, EventArgs e)
{
// 显示选中的行的数据
foreach (DataGridViewRow row in dataGridView1.SelectedRows)
{
if (!row.IsNewRow)
{
Person person = people[row.Index];
txtName.Text = person.Name;
txtAge.Text = person.Age.ToString();
}
}
}
}
```
在这个例子中,我们创建了一个Person类来表示人物数据。然后,我们创建了一个BindingList<Person>对象来存储人物数据,并将其绑定到DataGridView控件上。在添加、删除和修改数据时,我们直接操作BindingList<Person>对象即可。同时,我们使用SelectionChanged事件来显示选中的行的数据。
阅读全文
相关推荐
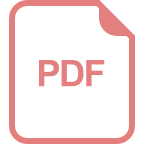
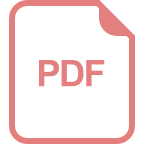
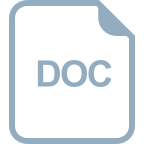
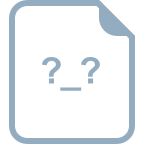
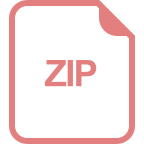
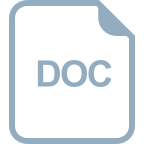
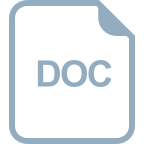
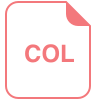
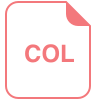






