python爬取淘宝商品信息
时间: 2023-07-21 12:03:28 浏览: 99
可以使用Python编写程序,利用cookies登录淘宝,并爬取商品信息。具体步骤如下:
1. 使用selenium模拟浏览器登录淘宝,并获取cookies信息。
2. 使用requests库发送请求,携带cookies信息,获取商品页面的HTML代码。
3. 使用正则表达式或BeautifulSoup库解析HTML代码,提取商品信息。
4. 将提取的商品信息保存到本地文件或数据库中。
需要注意的是,淘宝有反爬机制,需要设置合理的请求头和IP代理,避免被封禁。另外,爬取淘宝商品信息也需要遵守相关法律法规,不得用于商业用途。
相关问题
python爬取淘宝商品信息selenium
你可以使用Selenium来爬取淘宝商品信息。下面是一个简单的示例代码,演示了如何使用Selenium在Python中进行淘宝商品信息爬取:
```python
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
# 设置Chrome浏览器的驱动路径
driver_path = 'path/to/chromedriver'
# 初始化Chrome浏览器
options = webdriver.ChromeOptions()
options.add_argument('--headless') # 无头模式,不显示浏览器界面
driver = webdriver.Chrome(executable_path=driver_path, options=options)
# 打开淘宝商品页面
driver.get('https://www.taobao.com/')
# 定位搜索框并输入关键字
search_input = driver.find_element(By.CSS_SELECTOR, '#q')
search_input.send_keys('商品关键字')
# 点击搜索按钮
search_button = driver.find_element(By.CSS_SELECTOR, '.btn-search')
search_button.click()
# 等待搜索结果加载完成
WebDriverWait(driver, 10).until(EC.presence_of_element_located((By.CSS_SELECTOR, '.JIIxO.m-itemlist')))
# 解析搜索结果并提取商品信息
items = driver.find_elements(By.CSS_SELECTOR, '.JIIxO.m-itemlist .JIIxO.m-itemlist .JIIxO')
for item in items:
title = item.find_element(By.CSS_SELECTOR, '.title').text
price = item.find_element(By.CSS_SELECTOR, '.price').text
print(f'商品名称:{title},价格:{price}')
# 关闭浏览器
driver.quit()
```
请注意,这只是一个简单的示例代码,实际应用中可能需要更多的处理和错误处理。此外,淘宝网站有一些反爬虫机制,因此在实际使用时需要注意遵守相关规定,避免被封禁。
python爬取淘宝商品信息csdn
Python爬虫可以通过网络请求抓取淘宝商品信息,这里假设使用requests和BeautifulSoup库实现。首先,我们需要导入所需的库:
```
import requests
from bs4 import BeautifulSoup
```
然后,我们可以定义一个函数来获取淘宝商品信息:
```
def crawl_taobao(keyword):
url = 'https://search.taobao.com/search?q=' + keyword
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'
} # 模拟浏览器访问
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, 'html.parser')
items = soup.select('.J_MouserOnverReq')
for item in items:
title = item.select('.J_ClickStat')[0].text
price = item.select('.price')[0].text
shop = item.select('.shop')[0].text.strip()
print('商品名称:', title)
print('商品价格:', price)
print('商品店铺:', shop)
print('----------------------')
# 使用示例
crawl_taobao('手机')
```
上述代码中,我们首先构建了淘宝搜索的URL,然后发送请求并获取响应。接着,利用BeautifulSoup解析页面内容,找到商品的名称、价格和店铺信息,并进行打印输出。
需要注意的是,为了模拟浏览器的请求,我们设置了较为完整的User-Agent,这样可以避免遭到反爬虫的屏蔽。
执行上述代码,即可实现通过Python爬取淘宝商品信息。当然,更复杂的操作和数据处理都可以在上述代码的基础上进行拓展和优化。
阅读全文
相关推荐
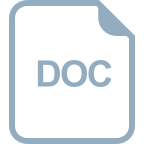
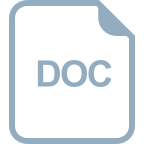
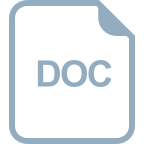
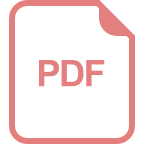


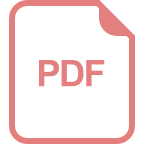


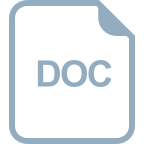
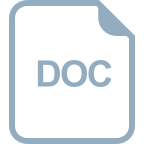
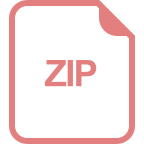
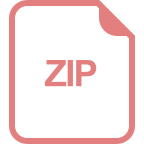
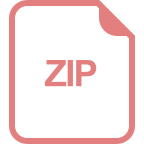