java读取yaml文件内容包括注释
时间: 2023-10-24 16:04:52 浏览: 38
Java读取YAML文件内容包括注释可以通过以下步骤实现:
1. 引入YAML解析库,如jyaml或snakeyaml。
2. 使用YAML解析库读取YAML文件内容。
3. 将读取到的内容存储到一个Map对象中,其中包含键值对和注释。
以下是一个示例代码:
```java
import org.yaml.snakeyaml.Yaml;
import org.yaml.snakeyaml.nodes.Node;
import org.yaml.snakeyaml.reader.StreamReader;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.InputStream;
import java.util.*;
public class YamlReader {
public static void main(String[] args) {
Yaml yaml = new Yaml();
try {
InputStream stream = new FileInputStream("test.yaml");
Map<String, Object> document = (Map<String, Object>) yaml.load(stream);
Map<String, List<String>> comments = extractComments(stream);
for (Map.Entry<String, Object> entry : document.entrySet()) {
String key = entry.getKey();
Object value = entry.getValue();
List<String> commentList = comments.get(key);
if (commentList != null) {
for (String comment : commentList) {
System.out.println(comment);
}
}
System.out.println(key + ": " + value);
}
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
private static Map<String, List<String>> extractComments(InputStream stream) {
Map<String, List<String>> comments = new HashMap<>();
try {
StreamReader reader = new StreamReader(stream);
while (reader.hasMore()) {
Node node = reader.peekNode();
if (node != null && node.getStartMark().getColumn() == 0 && node.getEndMark().getColumn() == 0) {
List<String> commentList = new ArrayList<>();
while (node != null && node.getStartMark().getColumn() == 0 && node.getEndMark().getColumn() == 0) {
commentList.add(reader.getNextLine());
node = reader.peekNode();
}
comments.put(node.getStartMark().getLine() + "", commentList);
} else {
reader.getNextLine();
}
}
} catch (Exception e) {
e.printStackTrace();
}
return comments;
}
}
```
在这个示例中,我们使用了SnakeYAML库来读取YAML文件内容,并使用extractComments方法从文件中提取注释。在主方法中,我们遍历读取到的内容,根据每个键值对的键从注释Map中获取注释,并将注释和值一起输出。
相关推荐
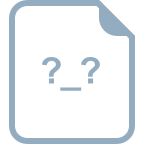
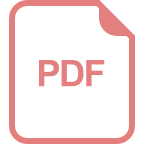
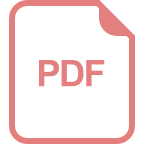
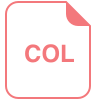
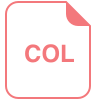
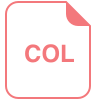
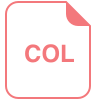









