把数组分批遍历,请给出csharp代码
时间: 2024-05-10 20:19:55 浏览: 49
Sure, here's some sample C# code for iterating over an array in batches:
```
int batchSize = 10;
int[] myArray = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15 };
int numberOfBatches = myArray.Length / batchSize;
for (int i = 0; i <= numberOfBatches; i++)
{
int startIndex = i * batchSize;
int endIndex = Math.Min(startIndex + batchSize, myArray.Length);
for (int j = startIndex; j < endIndex; j++)
{
Console.WriteLine(myArray[j]);
}
}
```
This code will iterate over an array of integers in batches of 10, printing each element to the console. If the length of the array is not divisible by the batch size, the final batch will contain the remaining elements.
相关问题
把数组分批遍历到一个新数组,请给出csharp代码
以下是分批遍历数组并生成新数组的 C# 代码:
```
public static T[] BatchedArray<T>(T[] sourceArray, int batchSize)
{
int numberOfBatches = (int)Math.Ceiling((double)sourceArray.Length / batchSize);
T[] resultArray = new T[numberOfBatches];
for (int i = 0; i < numberOfBatches; i++)
{
int currentIndex = i * batchSize;
T[] currentBatch = new T[Math.Min(batchSize, sourceArray.Length - currentIndex)];
for (int j = 0; j < currentBatch.Length; j++)
{
currentBatch[j] = sourceArray[currentIndex + j];
}
resultArray[i] = currentBatch;
}
return resultArray;
}
```
使用方法:
```
int[] sourceArray = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
int batchSize = 3;
int[][] resultArray = BatchedArray(sourceArray, batchSize);
// Output: {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}, {10}}
Console.WriteLine(string.Join(", ", resultArray.Select(batch => $"{{{string.Join(", ", batch)}}}")));
```
用webclient分批上传图片数据到服务器,要求用multipart/form-data格式上报
如果您需要使用 WebClient 来分批上传图片数据,并且要求使用 `multipart/form-data` 格式进行上报,可以按照以下步骤进行操作:
1. 将图片数据分割成较小的块。您可以使用 `File.ReadAllBytes` 方法将整个图片文件读取为字节数组,然后将字节数组分割成块。
2. 创建一个 `WebClient` 对象,并设置请求头的 `Content-Type` 为 `multipart/form-data`。代码示例如下:
```csharp
WebClient client = new WebClient();
client.Headers.Add("Content-Type", "multipart/form-data");
```
3. 遍历每个块,依次上传。对于每个块,您需要构建一个 `NameValueCollection` 对象,用于存储上传的表单数据。然后通过 `UploadValues` 方法上传该块的数据。代码示例如下:
```csharp
byte[] chunkData = // 从分割的块中获取数据
NameValueCollection formData = new NameValueCollection();
formData.Add("file", Convert.ToBase64String(chunkData)); // 将块中的数据转换为 Base64 字符串并添加到表单数据中
byte[] response = client.UploadValues("http://your-server-url", formData);
// 处理服务器的响应
```
4. 在服务器端接收数据时,您需要根据 `multipart/form-data` 的格式进行解析。具体解析方式取决于服务器端的编程语言和框架。一般情况下,服务器端会提供相应的工具或库来处理 `multipart/form-data` 格式的数据。
注意:在进行分块上传时,您需要确保服务器端能够正确地将这些块合并成完整的图片文件。服务器端可能需要提供相应的接口支持。
阅读全文
相关推荐
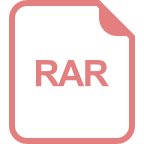
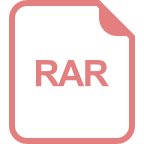
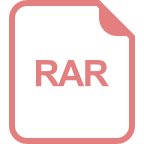
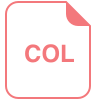
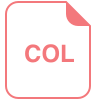
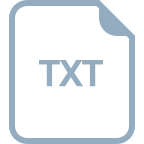
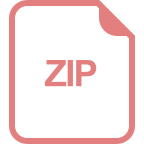
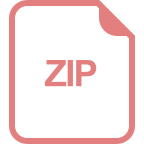
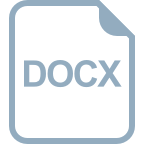
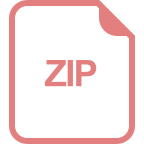
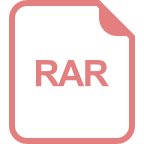
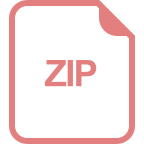
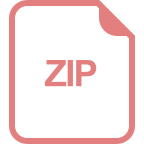
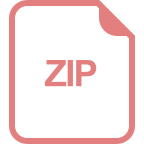
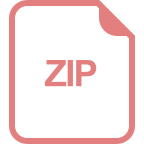