用C语言解决从键盘输入若干个学生的C语言成绩,以输入负数作为输入结束标记。用数组和函数实现求解最高分和将所有成绩降序排列的问题
时间: 2023-06-11 08:08:37 浏览: 278
可以使用以下代码实现:
```c
#include <stdio.h>
#define MAX_NUM 100
// 函数声明
void sort_desc(int arr[], int n);
int max_score(int arr[], int n);
int main() {
int scores[MAX_NUM];
int n = 0;
printf("请输入学生的成绩,以负数作为输入结束标记:\n");
// 读入成绩
while (1) {
int score;
scanf("%d", &score);
if (score < 0) {
break;
}
scores[n++] = score;
}
// 求最高分
int highest = max_score(scores, n);
printf("最高分为:%d\n", highest);
// 降序排列
sort_desc(scores, n);
printf("成绩降序排列为:");
for (int i = 0; i < n; i++) {
printf("%d ", scores[i]);
}
printf("\n");
return 0;
}
// 函数定义
void sort_desc(int arr[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = i + 1; j < n; j++) {
if (arr[i] < arr[j]) {
// 交换元素
int tmp = arr[i];
arr[i] = arr[j];
arr[j] = tmp;
}
}
}
}
int max_score(int arr[], int n) {
int max = arr[0];
for (int i = 1; i < n; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
```
在该代码中,使用数组 `scores` 存储输入的成绩。通过 `while` 循环不断读取输入的成绩,直到读取到负数为止。然后调用 `max_score` 函数求出最高分,调用 `sort_desc` 函数将成绩降序排列。最后输出结果。
`max_score` 函数接受一个整型数组和数组长度作为参数,返回数组中最大的值。该函数遍历数组,找到最大的值并返回。
`sort_desc` 函数接受一个整型数组和数组长度作为参数,将数组按降序排列。该函数使用双重循环,比较相邻的两个元素,如果前一个元素比后一个元素小,则交换两个元素的值。
阅读全文
相关推荐
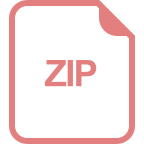




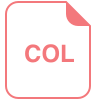
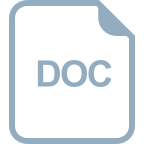
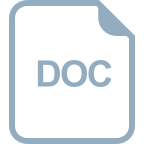
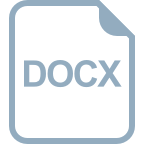
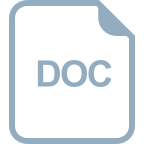
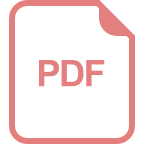
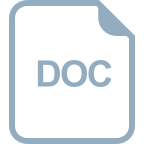
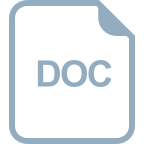
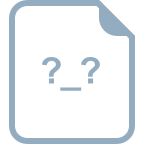
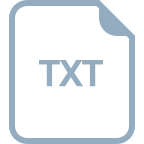