尝试使用文件选择器javax.swing.JFileChooser类,并分别用 showOpenDialog(Component parent) showSaveDialog(Component parent) 方法弹出“打开文件”和“保存文件”两种模式。
时间: 2024-03-18 12:41:32 浏览: 84
好的,我可以为您提供代码示例。
打开文件模式:
```java
import javax.swing.*;
import java.awt.*;
public class FileChooserDemo {
public static void main(String[] args) {
JFrame frame = new JFrame("File Chooser Demo");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 300);
frame.setLocationRelativeTo(null);
JButton openButton = new JButton("Open File");
openButton.addActionListener(e -> {
JFileChooser fileChooser = new JFileChooser();
int result = fileChooser.showOpenDialog(frame);
if (result == JFileChooser.APPROVE_OPTION) {
String filePath = fileChooser.getSelectedFile().getAbsolutePath();
JOptionPane.showMessageDialog(frame, "You selected file: " + filePath);
} else {
JOptionPane.showMessageDialog(frame, "You did not select any file.");
}
});
JPanel panel = new JPanel();
panel.add(openButton);
frame.getContentPane().add(panel, BorderLayout.CENTER);
frame.setVisible(true);
}
}
```
保存文件模式:
```java
import javax.swing.*;
import java.awt.*;
public class FileChooserDemo {
public static void main(String[] args) {
JFrame frame = new JFrame("File Chooser Demo");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 300);
frame.setLocationRelativeTo(null);
JButton saveButton = new JButton("Save File");
saveButton.addActionListener(e -> {
JFileChooser fileChooser = new JFileChooser();
int result = fileChooser.showSaveDialog(frame);
if (result == JFileChooser.APPROVE_OPTION) {
String filePath = fileChooser.getSelectedFile().getAbsolutePath();
JOptionPane.showMessageDialog(frame, "You selected file: " + filePath);
} else {
JOptionPane.showMessageDialog(frame, "You did not select any file.");
}
});
JPanel panel = new JPanel();
panel.add(saveButton);
frame.getContentPane().add(panel, BorderLayout.CENTER);
frame.setVisible(true);
}
}
```
这两个示例代码中,我们都创建了一个 JFrame 对象作为父组件,然后在按钮的 ActionListener 中创建了一个 JFileChooser 对象,并调用了相应的方法弹出文件选择对话框。最后根据用户的选择结果,我们可以得到所选文件的路径。
阅读全文
相关推荐
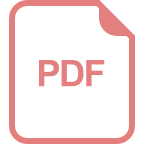



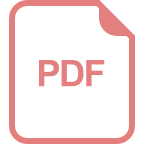
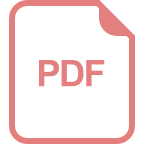
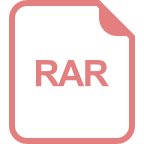
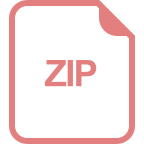
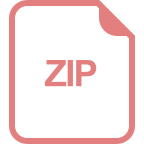
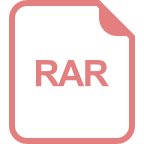
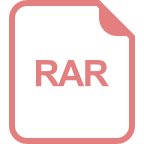
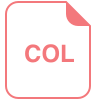




