java实现决策树代码
时间: 2023-06-07 10:07:23 浏览: 104
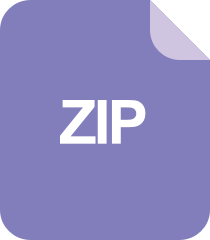
基于java实现的决策树代码
我可以为您提供一个基本的 Java 决策树实现代码,但由于您没有提供更具体的要求,所以我将为您提供一个常规代码的示例:
```
public class DecisionTree {
static class TreeNode {
String data;
TreeNode left, right;
TreeNode(String data) {
this.data = data;
left = null;
right = null;
}
}
public static void main(String[] args) {
TreeNode root = new TreeNode("Is it sunny outside?");
root.left = new TreeNode("Do you want to go outside?");
root.right = new TreeNode("Stay indoors and read a book.");
root.left.left = new TreeNode("Is the temperature too hot?");
root.left.right = new TreeNode("Go for a walk.");
root.left.left.left = new TreeNode("Stay indoors and keep cool.");
root.left.left.right = new TreeNode("Enjoy the weather.");
System.out.println("Decision tree created.");
}
}
```
这个简单的示例创建了一个决策树来决定一个人是否应该外出。其中,根节点询问是否阳光明媚,是则询问是否想外出,不是则建议呆室内看书。若想外出,则继续询问温度,高于宜人范围就呆在室内,并给出保持凉爽的建议。否则,就告诉人们外出并享受阳光。
阅读全文
相关推荐
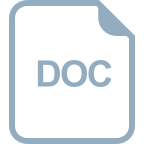
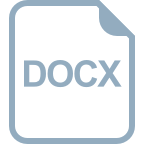
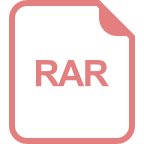
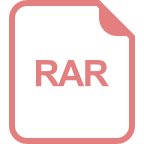
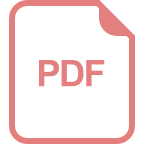
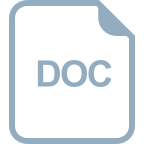
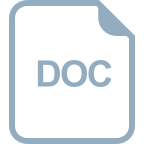
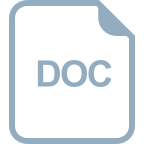
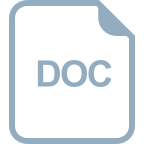
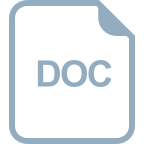




